طرق JavaScript والمزيد. دورة JavaScript للجزء 9 من المستوى المبتدئ إلى المتقدم في 10 منشورات مدونة
نشرت: 2021-11-09هذا جزء 9 من سلسلة منشورات مدونة JavaScript التي ستأخذك من المبتدئين إلى المتقدمين. هذه المرة سنستكشف الموضوعات التالية: طرق JavaScript ، وقيم المعلمات الافتراضية ، وكائن التاريخ في JavaScript ووظيفة السهم. بنهاية هذه السلسلة ، ستعرف جميع الأساسيات التي تحتاج إلى معرفتها لبدء الترميز في JavaScript. إذا لم تكن قد قرأت منشور المدونة السابق حول كائنات JavaScript ، فيمكنك القيام بذلك هنا. بدون مزيد من اللغط ، لنبدأ بالدرس التاسع.
طرق JavaScript والمزيد - جدول المحتويات:
- قيم المعلمات الافتراضية
- كائن التاريخ في JavaScript
- طريقة الخريطة ()
- طريقة التصفية ()
- دالة السهم
لقد رأينا حتى الآن العديد من المفاهيم والموضوعات في JavaScript ، ولكن لا تزال هناك بعض المفاهيم الشائعة الاستخدام التي لم نكتشفها. في هذا البرنامج التعليمي ، سنرى ما يدور حوله كل شيء. الأول هو قيم المعلمات الافتراضية في JavaScript.
قيم المعلمات الافتراضية
تُستخدم الوظائف بشكل شائع للغاية في البرمجة ، وعندما يتم استخدام شيء ما ، غالبًا ما لا توجد أطر عمل مثل React التي تستفيد من وظائف JavaScript ، ولكن هناك أيضًا تحسينات أخرى تم تطويرها للحصول على المزيد من وظائف JavaScript. واحدة من الميزات الرئيسية التي لدينا في الوظائف تسمى قيم المعلمات الافتراضية. تسمح لنا المعلمات الافتراضية بكتابة أكواد أكثر أمانًا يمكنها عمل افتراضات آمنة حول مدخلات المستخدم. يفيد هذا المستخدم أيضًا من خلال تزويده بإعدادات افتراضية تجعل من السهل الاختيار من بين خياراته أيضًا. دعونا نرى بعض الأمثلة على ذلك.
// assume you are developing a website for a coffee shop // and most people want to get a medium size americano // knowing this you want to make it easir for people to // order their coffee with less hustle function orderCoffee(typeOfCoffee="americano", sizeOfCoffee="medium", numberOfCoffee=1){ return (numberOfCoffee + " " + sizeOfCoffee + " size " + typeOfCoffee + " is being prepared. "); } console.log("Default order:"); console.log(orderCoffee()); // this way, when an average customer orders online, // it will be much easier for them to order their daily coffee // so much so that they will be able to order it with a single button // alternatively people can also customize their coffee // by changing the inputs console.log("Custom order:"); console.log(orderCoffee("latte", "large", 2)); console.log("Another custom order:"); console.log(orderCoffee("macchiato", "small", 1)); // it is also possible to change only part of the inputs // and leverage the default parameters // for the rest of the input fields console.log("Partially customized order:"); console.log(orderCoffee("iced coffee"));
يمنحنا تشغيل الكود أعلاه المخرجات التالية:
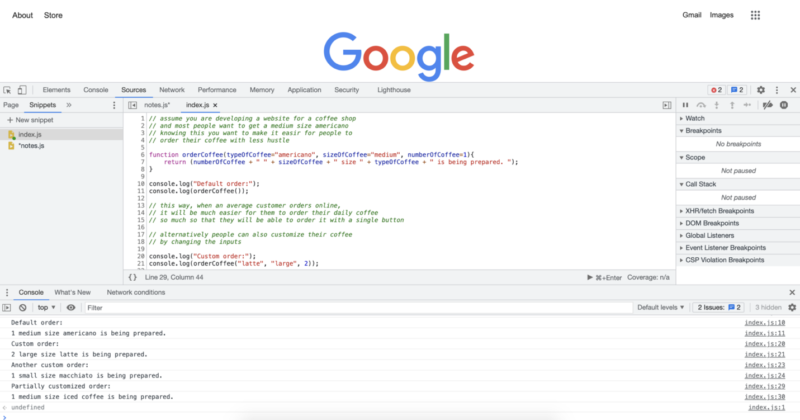
كائن التاريخ في JavaScript
يتم استخدام كائن التاريخ في JavaScript بشكل شائع ، خاصة في تطوير الويب. يمكننا استخدام كائن التاريخ لأداء وظائف حساسة للوقت مثل تغيير إعدادات العرض إلى الوضع الداكن أو وضع الإضاءة أو أي وضع آخر قد يفضله المستخدم. يمكننا أيضًا استخدام معلومات التاريخ حسب الحاجة داخل المشروع الذي نعمل عليه. فيما يلي بعض الأمثلة على كائن التاريخ قيد التشغيل:
// the first step is to create a date object instance // we cna do this by writing the following and // setting it to a variable or constant of our choice let today = new Date(); console.log("We are at year: " + today.getFullYear()); console.log("We can also get month in number:"); console.log(today.getMonth()); console.log("Also we can get the hour of the day like this:"); console.log(today.getHours()); console.log("We can also get the exact minutes along with the seconds"); console.log(today.getMinutes()); console.log(today.getSeconds()); // once we have these numbers we can use them as we like // if we want we can display them or make decisions based on them. // if we want to display month with a name // rather than a number, we can also achieve that // with the following const months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]; console.log("We are at the month of " + months[today.getMonth()]); // what we just did was to create an array to store month names // and then choose the correct month using an index value // provided by the .getMonth() method. // if we wanted to turn on dark mode after 8 pm, // we could do that with the following code // one of the first thing we should remember is that // hours are given in the 24 hour format // that means 8pm will mean 20 as hours // we can also use a short hand way // and combine the new date object creation // with the get hours method let timeOfDay = new Date().getHours(); if (timeOfDay >= 20) { console.log("Turning on Dark Mode..."); } else { console.log("Do not turn on the Dark Mode"); } // since current time is over 8pm, // in this case we expect to get turn on Dark Mode. // which is also the reuslt we get as we can see from // the console output.
سيعطينا تشغيل الكود أعلاه سجلات وحدة التحكم التالية:
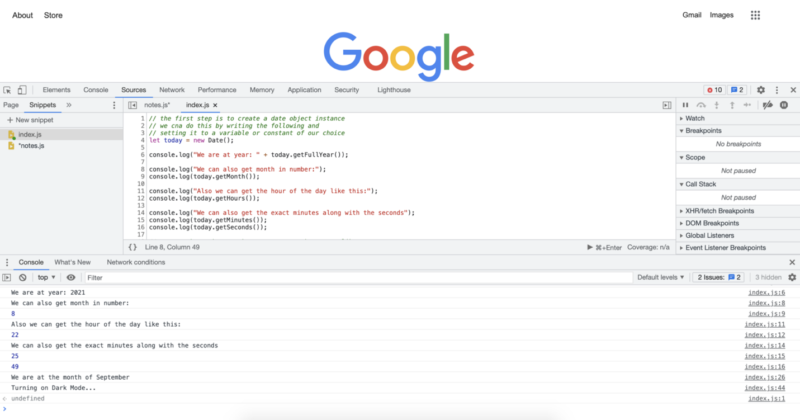
طريقة الخريطة ()
طريقة الخريطة هي طريقة مفيدة للغاية يمكن أن توفر لك العديد من سطور التعليمات البرمجية ، واعتمادًا على كيفية استخدامها يمكن أن تجعل الكود الخاص بك أكثر نظافة. يستبدل بشكل أساسي استخدام حلقة for عند استخدامها للتكرار فوق مصفوفة. فيما يلي بعض الأمثلة لتعيين طريقة ().
// lets create an array we will use for mapping let someNumbers = [1, 2, 3, 4, 5]; // lets also create the functions we will // provide to the map method function doubleNums(num1){ return num1 * 2; } function squareNums(num1){ return num1 * num1; } function add100(num1){ return num1 + 100; } console.log("Doubled numbers array:"); console.log(someNumbers.map(doubleNums)); console.log("Squared numbers array:"); console.log(someNumbers.map(squareNums)); console.log("100 added to each of the element in the umbers array:"); console.log(someNumbers.map(add100)); // map() method will loop over each of the // items in a given array and apply the // provided function // note that we do not include paranthesis // after the function names, this would call the function // instead we pass the function name, // and map() method calls them when it needs to
سيعطينا تشغيل الكود أعلاه سجلات وحدة التحكم التالية:

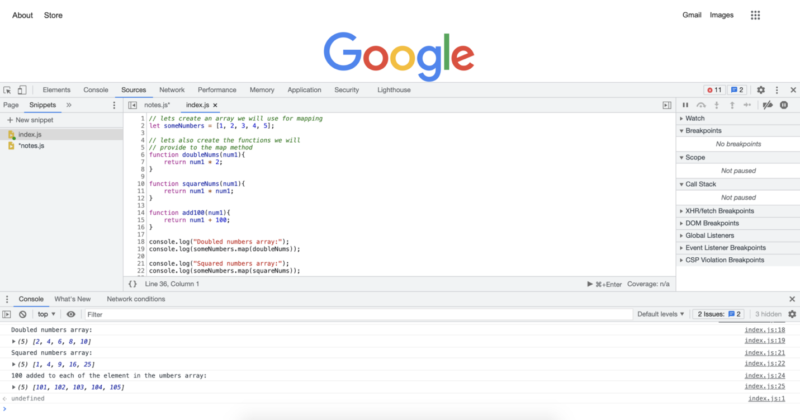
طريقة التصفية ()
تعد طريقة filter () جنبًا إلى جنب مع طريقة map () من طرق JavaScript الشائعة جدًا. إنها تشبه طريقة الخريطة () التي رأيناها للتو. باستخدام طريقة map () ، يمكننا تمرير أي دالة ، ويتم تطبيق هذه الوظيفة على كل عنصر من عناصر المصفوفة. باستخدام طريقة filter () ، سنمرر معايير مرشح وستقوم طريقة التصفية بتكرار جميع العناصر في المصفوفة وستعيد مصفوفة جديدة مع بقاء العناصر التي تمرر المعايير فقط. دعونا نرى بعض الأمثلة على ذلك:
// lets first create an array // to apply the filter() method let someNumbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21]; function checkEven(num1){ if (num1 % 2 == 0){ return num1; } } function checkOdd(num1){ if (num1 % 2 == 1){ return num1; } } function over13(num1){ if (num1 > 13){ return num1; } } function divisibleByFive(num){ if (num % 5 == 0){ return num; } } console.log("Even numbers from the list:"); console.log(someNumbers.filter(checkEven)); console.log("Odd numbers from the list:"); console.log(someNumbers.filter(checkOdd)); console.log("Numbers over 13 from the array:"); console.log(someNumbers.filter(over13)); console.log("Numbers divisible by 5 from the array:"); console.log(someNumbers.filter(divisibleByFive));سيعطينا تشغيل الكود أعلاه سجلات وحدة التحكم التالية:
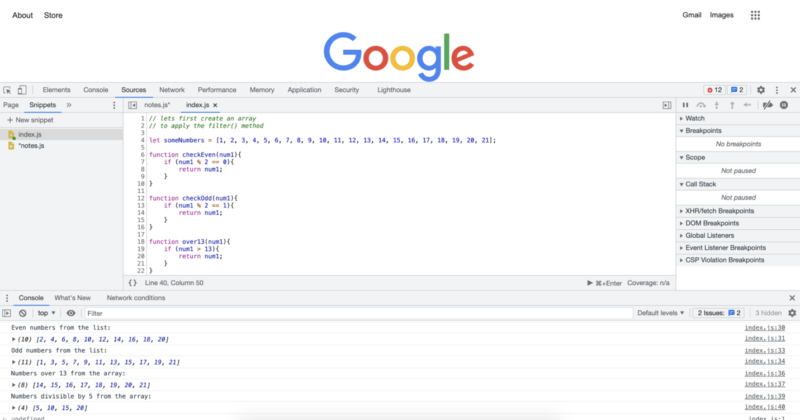
دالة السهم
تذكر عندما قلنا أن الوظائف شائعة جدًا في JavaScript وهناك العديد من التحسينات التي تم إجراؤها عليها للحصول على كود أكثر أداءً أو نظيفًا؟ حسنًا ، وظائف الأسهم هي واحدة منها. يشار أحيانًا إلى وظائف الأسهم أيضًا باسم سهم الدهون. إنها توفر طريقة أقصر بكثير لكتابة وظائفك. كما يتم استخدامها بشكل شائع مع طرق JavaScript التي رأيناها للتو. دعونا نراهم مع بعض الأمثلة:
// JavaScript provides multiple levels of // code shortening with arrow functions depending on your exact code // Essantially the longest way we can write a function is // the way we always write them without using the arrow functions // lets start with an array to apply the arrow functions let someNumbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21]; console.log("Original Array:"); console.log(someNumbers); // in the previous examples we have applied many functions // after creating them as regular named functions // In this example we will apply the exact transformations // so that you can see the both extremes in code lenght // double every number in the array: console.log("Double every number in the array:") console.log(someNumbers.map(num => num * 2)); // square every number in the array: console.log("Square every number in the array:") console.log(someNumbers.map(num => num * num)); // add 100 to every number in the array: console.log("Add 100 to every number in the array:") console.log(someNumbers.map(num => num + 100)); // Only keep the even numbers in the array: console.log("Only keep the even numbers in the array:") console.log(someNumbers.filter(num => num % 2 == 0)); // Only keep the odd numbers in the array: console.log("Only keep the odd numbers in the array:") console.log(someNumbers.filter(num => num % 2 == 1)); // Only keep the numbers that are evenly divisible by 5: console.log("Only keep the numbers that are evenly divisible by 5:") console.log(someNumbers.filter(num => num % 5 == 0)); // Only keep the numbers that are over 13: console.log("Only keep the numbers that are over 13:") console.log(someNumbers.filter(num => num > 13));
سيعطينا تشغيل الكود أعلاه سجلات وحدة التحكم التالية:
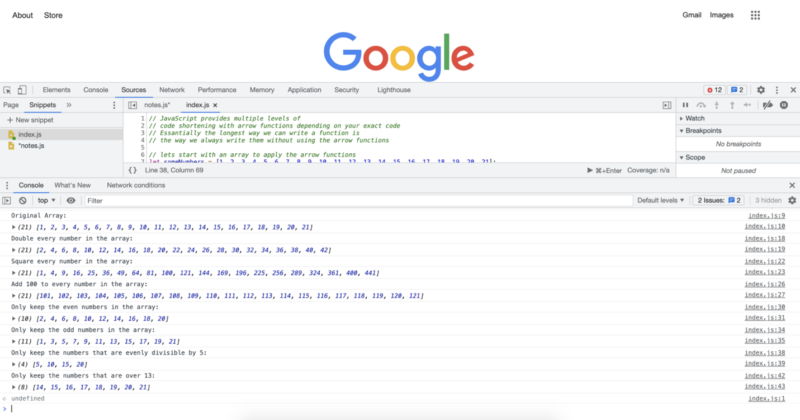
في البرنامج التعليمي التالي ، سيكون لدينا نظرة عامة على ما رأيناه ونرى ما هو التالي.
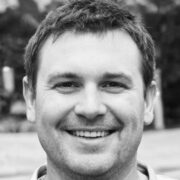
المؤلف: روبرت ويتني
خبير JavaScript ومدرب يدرب أقسام تكنولوجيا المعلومات. هدفه الرئيسي هو رفع مستوى إنتاجية الفريق من خلال تعليم الآخرين كيفية التعاون الفعال أثناء البرمجة.
دورة JavaScript من المبتدئين إلى المتقدمين في 10 منشورات مدونة:
- كيف تبدأ الترميز في JavaScript؟
- أساسيات JavaScript
- المتغيرات وأنواع البيانات المختلفة في JavaScript
- المقتطفات وهياكل التحكم
- بينما الحلقات والحلقات
- مجموعة جافا
- وظائف JavaScript
- كائنات جافا سكريبت
- طرق JavaScript والمزيد
- ملخص دورة JavaScript