JavaScript-Methoden und mehr. Teil 9 JavaScript-Kurs vom Anfänger bis zum Fortgeschrittenen in 10 Blogbeiträgen
Veröffentlicht: 2021-11-09Dies ist Teil 9 der JavaScript-Blogpost-Serie, die Sie vom Anfänger bis zum Fortgeschrittenen führt. Dieses Mal untersuchen wir die folgenden Themen: JavaScript-Methoden, Standardparameterwerte, das Date-Objekt in JavaScript und die Pfeilfunktion. Am Ende dieser Serie kennen Sie alle Grundlagen, die Sie wissen müssen, um mit dem Programmieren in JavaScript zu beginnen. Wenn Sie den vorherigen Blogbeitrag über JavaScript-Objekte noch nicht gelesen haben, können Sie dies hier tun. Beginnen wir ohne weiteres mit dem neunten Tutorial.
JavaScript-Methoden und mehr – Inhaltsverzeichnis:
- Standardparameterwerte
- Das Date-Objekt in JavaScript
- Map()-Methode
- Filter()-Methode
- Pfeilfunktion
Bis jetzt haben wir viele Konzepte und Themen in JavaScript gesehen, aber es gibt immer noch einige häufig verwendete, die wir nicht entdeckt haben. In diesem Tutorial werden wir sehen, worum es bei ihnen geht. Der erste sind die Standardparameterwerte in JavaScript.
Standardparameterwerte
Funktionen werden sehr häufig in der Programmierung verwendet, und wenn etwas so oft verwendet wird, gibt es nicht nur Frameworks wie React, die JavaScript-Funktionen nutzen, sondern es wurden sogar weitere Optimierungen entwickelt, um noch mehr aus JavaScript-Funktionen herauszuholen. Eines der Hauptmerkmale, die wir in Funktionen haben, heißt Standardparameterwerte. Standardparameter ermöglichen es uns, sicherere Codes zu schreiben, die sichere Annahmen über die Benutzereingabe treffen können. Dies kommt auch dem Benutzer zugute, indem ihm eine Standardeinstellung bereitgestellt wird, die es ebenfalls einfacher machen kann, aus seinen Optionen auszuwählen. Sehen wir uns dazu einige Beispiele an.
// assume you are developing a website for a coffee shop // and most people want to get a medium size americano // knowing this you want to make it easir for people to // order their coffee with less hustle function orderCoffee(typeOfCoffee="americano", sizeOfCoffee="medium", numberOfCoffee=1){ return (numberOfCoffee + " " + sizeOfCoffee + " size " + typeOfCoffee + " is being prepared. "); } console.log("Default order:"); console.log(orderCoffee()); // this way, when an average customer orders online, // it will be much easier for them to order their daily coffee // so much so that they will be able to order it with a single button // alternatively people can also customize their coffee // by changing the inputs console.log("Custom order:"); console.log(orderCoffee("latte", "large", 2)); console.log("Another custom order:"); console.log(orderCoffee("macchiato", "small", 1)); // it is also possible to change only part of the inputs // and leverage the default parameters // for the rest of the input fields console.log("Partially customized order:"); console.log(orderCoffee("iced coffee"));
Wenn Sie den obigen Code ausführen, erhalten Sie die folgende Ausgabe:
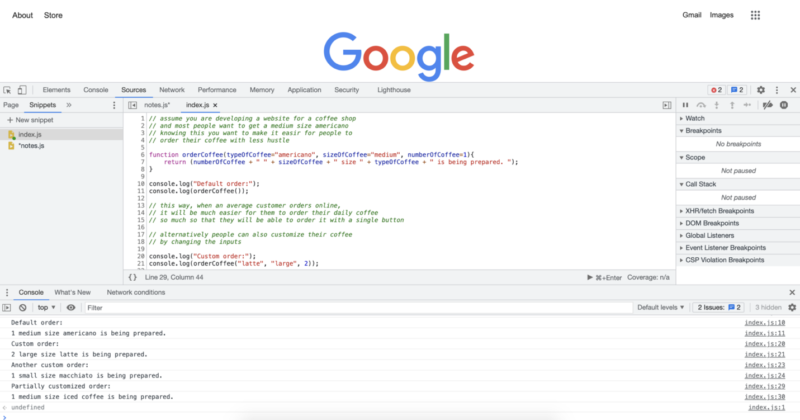
Das Date-Objekt in JavaScript
Das Date-Objekt in JavaScript wird ziemlich häufig verwendet, insbesondere in der Webentwicklung. Wir können das Date-Objekt verwenden, um zeitkritische Funktionen auszuführen, wie z. B. das Ändern der Anzeigeeinstellungen in den dunklen Modus, den hellen Modus oder einen anderen Modus, den der Benutzer möglicherweise bevorzugt. Wir können die Datumsinformationen auch nach Bedarf innerhalb des Projekts verwenden, an dem wir arbeiten. Hier sind einige Beispiele für das Date-Objekt in Aktion:
// the first step is to create a date object instance // we cna do this by writing the following and // setting it to a variable or constant of our choice let today = new Date(); console.log("We are at year: " + today.getFullYear()); console.log("We can also get month in number:"); console.log(today.getMonth()); console.log("Also we can get the hour of the day like this:"); console.log(today.getHours()); console.log("We can also get the exact minutes along with the seconds"); console.log(today.getMinutes()); console.log(today.getSeconds()); // once we have these numbers we can use them as we like // if we want we can display them or make decisions based on them. // if we want to display month with a name // rather than a number, we can also achieve that // with the following const months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]; console.log("We are at the month of " + months[today.getMonth()]); // what we just did was to create an array to store month names // and then choose the correct month using an index value // provided by the .getMonth() method. // if we wanted to turn on dark mode after 8 pm, // we could do that with the following code // one of the first thing we should remember is that // hours are given in the 24 hour format // that means 8pm will mean 20 as hours // we can also use a short hand way // and combine the new date object creation // with the get hours method let timeOfDay = new Date().getHours(); if (timeOfDay >= 20) { console.log("Turning on Dark Mode..."); } else { console.log("Do not turn on the Dark Mode"); } // since current time is over 8pm, // in this case we expect to get turn on Dark Mode. // which is also the reuslt we get as we can see from // the console output.
Wenn Sie den obigen Code ausführen, erhalten Sie die folgenden Konsolenprotokolle:
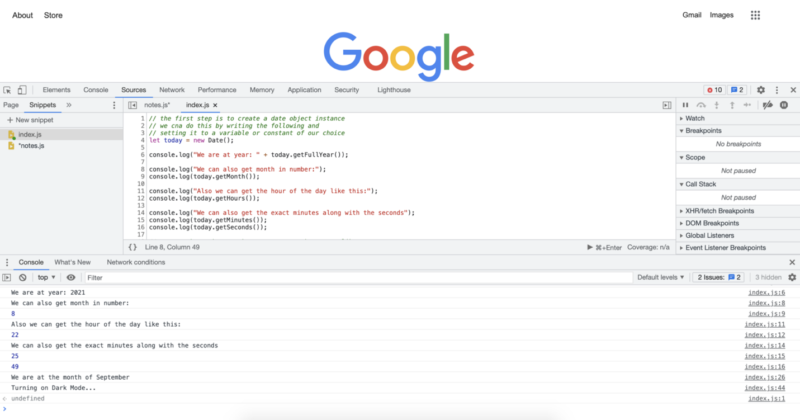
Map()-Methode
Die Map-Methode ist eine sehr nützliche Methode, mit der Sie viele Codezeilen einsparen können, und je nachdem, wie Sie sie verwenden, kann Ihr Code viel sauberer werden. Es ersetzt im Wesentlichen die Verwendung einer for-Schleife, wenn Sie damit ein Array durchlaufen. Hier sind einige Beispiele für die Methode map().
// lets create an array we will use for mapping let someNumbers = [1, 2, 3, 4, 5]; // lets also create the functions we will // provide to the map method function doubleNums(num1){ return num1 * 2; } function squareNums(num1){ return num1 * num1; } function add100(num1){ return num1 + 100; } console.log("Doubled numbers array:"); console.log(someNumbers.map(doubleNums)); console.log("Squared numbers array:"); console.log(someNumbers.map(squareNums)); console.log("100 added to each of the element in the umbers array:"); console.log(someNumbers.map(add100)); // map() method will loop over each of the // items in a given array and apply the // provided function // note that we do not include paranthesis // after the function names, this would call the function // instead we pass the function name, // and map() method calls them when it needs to
Wenn Sie den obigen Code ausführen, erhalten Sie die folgenden Konsolenprotokolle:

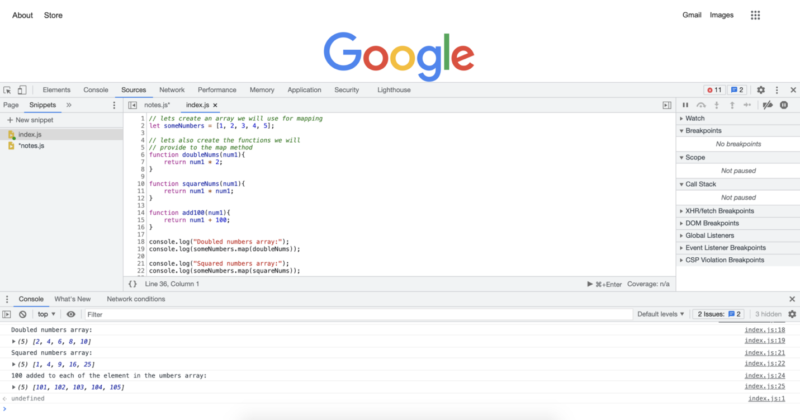
Filter()-Methode
Die filter()-Methode zusammen mit der map()-Methode sind beides recht verbreitete JavaScript-Methoden. Sie sind der gerade gesehenen Methode map() sehr ähnlich. Mit der Methode map() können wir jede Funktion übergeben, und diese Funktion wird auf jedes der Elemente in einem Array angewendet. Mit der filter()-Methode übergeben wir ein Filterkriterium und die filter-Methode durchläuft alle Elemente in einem Array und gibt ein neues Array zurück, in dem nur die Elemente verbleiben, die die Kriterien erfüllen. Sehen wir uns dazu einige Beispiele an:
// lets first create an array // to apply the filter() method let someNumbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21]; function checkEven(num1){ if (num1 % 2 == 0){ return num1; } } function checkOdd(num1){ if (num1 % 2 == 1){ return num1; } } function over13(num1){ if (num1 > 13){ return num1; } } function divisibleByFive(num){ if (num % 5 == 0){ return num; } } console.log("Even numbers from the list:"); console.log(someNumbers.filter(checkEven)); console.log("Odd numbers from the list:"); console.log(someNumbers.filter(checkOdd)); console.log("Numbers over 13 from the array:"); console.log(someNumbers.filter(over13)); console.log("Numbers divisible by 5 from the array:"); console.log(someNumbers.filter(divisibleByFive));Wenn Sie den obigen Code ausführen, erhalten Sie die folgenden Konsolenprotokolle:
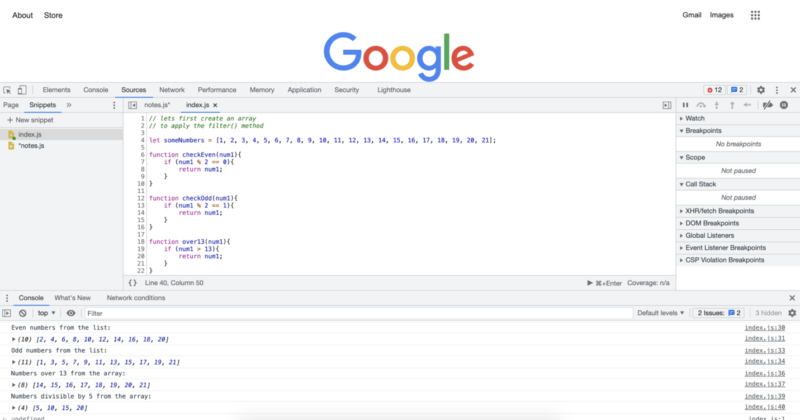
Pfeilfunktion
Erinnern Sie sich, als wir sagten, dass Funktionen in JavaScript sehr verbreitet sind und viele Optimierungen an ihnen vorgenommen werden, um noch leistungsfähigeren oder saubereren Code zu erhalten? Nun, Pfeilfunktionen sind eine davon. Pfeilfunktionen werden manchmal auch als fetter Pfeil bezeichnet. Sie bieten im Wesentlichen eine viel kürzere Möglichkeit, Ihre Funktionen zu schreiben. Sie werden auch sehr häufig mit den JavaScript-Methoden verwendet, die wir gerade gesehen haben. Sehen wir sie uns mit einigen Beispielen an:
// JavaScript provides multiple levels of // code shortening with arrow functions depending on your exact code // Essantially the longest way we can write a function is // the way we always write them without using the arrow functions // lets start with an array to apply the arrow functions let someNumbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21]; console.log("Original Array:"); console.log(someNumbers); // in the previous examples we have applied many functions // after creating them as regular named functions // In this example we will apply the exact transformations // so that you can see the both extremes in code lenght // double every number in the array: console.log("Double every number in the array:") console.log(someNumbers.map(num => num * 2)); // square every number in the array: console.log("Square every number in the array:") console.log(someNumbers.map(num => num * num)); // add 100 to every number in the array: console.log("Add 100 to every number in the array:") console.log(someNumbers.map(num => num + 100)); // Only keep the even numbers in the array: console.log("Only keep the even numbers in the array:") console.log(someNumbers.filter(num => num % 2 == 0)); // Only keep the odd numbers in the array: console.log("Only keep the odd numbers in the array:") console.log(someNumbers.filter(num => num % 2 == 1)); // Only keep the numbers that are evenly divisible by 5: console.log("Only keep the numbers that are evenly divisible by 5:") console.log(someNumbers.filter(num => num % 5 == 0)); // Only keep the numbers that are over 13: console.log("Only keep the numbers that are over 13:") console.log(someNumbers.filter(num => num > 13));
Wenn Sie den obigen Code ausführen, erhalten Sie die folgenden Konsolenprotokolle:
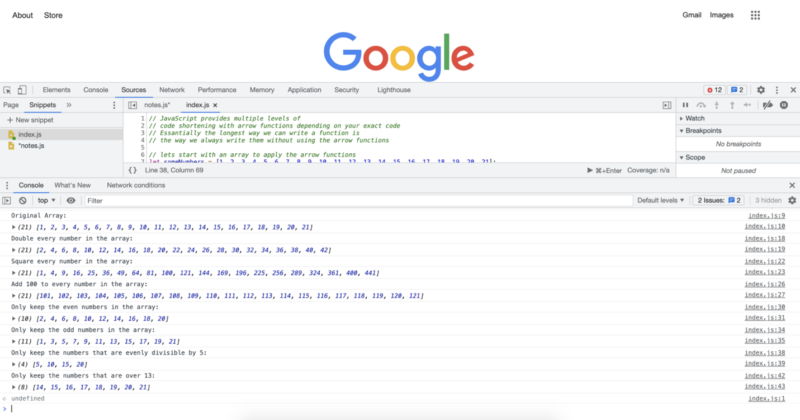
Im nächsten Tutorial werden wir einen Überblick über das Gesehene haben und sehen, was als nächstes kommt.
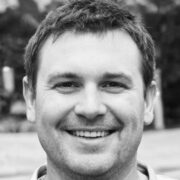
Autor: Robert Whitney
JavaScript-Experte und Ausbilder, der IT-Abteilungen coacht. Sein Hauptziel ist es, die Teamproduktivität zu steigern, indem er anderen beibringt, wie man beim Programmieren effektiv zusammenarbeitet.
JavaScript-Kurs vom Anfänger bis zum Fortgeschrittenen in 10 Blogbeiträgen:
- Wie fange ich mit dem Programmieren in JavaScript an?
- JavaScript-Grundlagen
- Variablen und verschiedene Datentypen in JavaScript
- Snippets und Kontrollstrukturen
- While-Schleifen und For-Schleifen
- Java-Array
- JavaScript-Funktionen
- JavaScript-Objekte
- JavaScript-Methoden und mehr
- Zusammenfassung des JavaScript-Kurses