JavaScript メソッドなど。 第9回 10回のブログ投稿で初心者から上級者までのJavaScriptコース
公開: 2021-11-09これは、初心者から上級者までを対象とした JavaScript ブログ投稿シリーズのパート 9 です。 今回は、JavaScript メソッド、デフォルトのパラメーター値、JavaScript の Date オブジェクト、アロー関数などのトピックについて説明します。 このシリーズの終わりまでに、JavaScript でコーディングを開始するために知っておく必要があるすべての基本を理解できます。 JavaScript オブジェクトに関する以前のブログ投稿をまだ読んでいない場合は、ここで読むことができます。 早速、9 番目のチュートリアルを始めましょう。
JavaScript メソッドなど – 目次:
- デフォルトのパラメータ値
- JavaScript の Date オブジェクト
- Map() メソッド
- Filter() メソッド
- アロー機能
これまで、JavaScript の多くの概念とトピックを見てきましたが、まだ発見されていない一般的に使用されるものがいくつかあります。 このチュートリアルでは、それらが何であるかを見ていきます。 1 つ目は、JavaScript のデフォルトのパラメーター値です。
デフォルトのパラメータ値
関数はプログラミングで非常に一般的に使用され、何かが使用される場合、JavaScript 関数を活用する React のようなフレームワークだけでなく、JavaScript 関数をさらに活用するためにさらに最適化が開発されています。 関数の主要な機能の 1 つは、既定のパラメーター値と呼ばれます。 デフォルトのパラメーターを使用すると、ユーザー入力について安全な推定を行うことができる、より安全なコードを書くことができます。 これは、オプションからの選択を容易にするデフォルト設定を提供することで、ユーザーにとってもメリットがあります。 その例をいくつか見てみましょう。
// assume you are developing a website for a coffee shop // and most people want to get a medium size americano // knowing this you want to make it easir for people to // order their coffee with less hustle function orderCoffee(typeOfCoffee="americano", sizeOfCoffee="medium", numberOfCoffee=1){ return (numberOfCoffee + " " + sizeOfCoffee + " size " + typeOfCoffee + " is being prepared. "); } console.log("Default order:"); console.log(orderCoffee()); // this way, when an average customer orders online, // it will be much easier for them to order their daily coffee // so much so that they will be able to order it with a single button // alternatively people can also customize their coffee // by changing the inputs console.log("Custom order:"); console.log(orderCoffee("latte", "large", 2)); console.log("Another custom order:"); console.log(orderCoffee("macchiato", "small", 1)); // it is also possible to change only part of the inputs // and leverage the default parameters // for the rest of the input fields console.log("Partially customized order:"); console.log(orderCoffee("iced coffee"));
上記のコードを実行すると、次の出力が得られます。
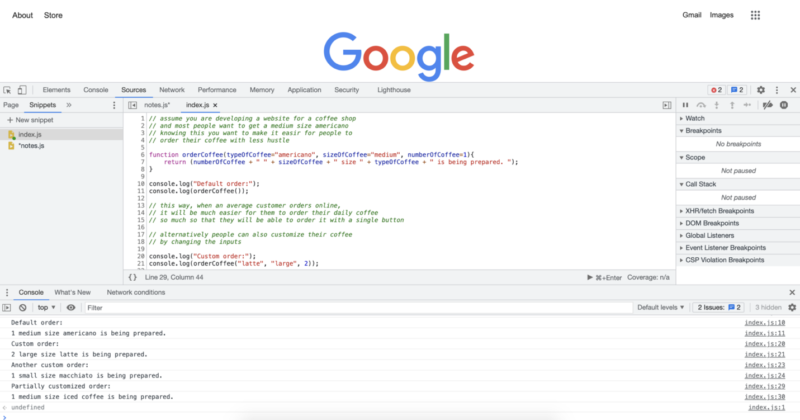
JavaScript の Date オブジェクト
JavaScript の Date オブジェクトは、特に Web 開発でよく使用されます。 Date オブジェクトを使用して、表示設定をダーク モード、ライト モード、またはユーザーが好むその他のモードに変更するなど、時間に依存する機能を実行できます。 作業中のプロジェクト内で必要に応じて日付情報を使用することもできます。 以下は、動作中の Date オブジェクトの例です。
// the first step is to create a date object instance // we cna do this by writing the following and // setting it to a variable or constant of our choice let today = new Date(); console.log("We are at year: " + today.getFullYear()); console.log("We can also get month in number:"); console.log(today.getMonth()); console.log("Also we can get the hour of the day like this:"); console.log(today.getHours()); console.log("We can also get the exact minutes along with the seconds"); console.log(today.getMinutes()); console.log(today.getSeconds()); // once we have these numbers we can use them as we like // if we want we can display them or make decisions based on them. // if we want to display month with a name // rather than a number, we can also achieve that // with the following const months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]; console.log("We are at the month of " + months[today.getMonth()]); // what we just did was to create an array to store month names // and then choose the correct month using an index value // provided by the .getMonth() method. // if we wanted to turn on dark mode after 8 pm, // we could do that with the following code // one of the first thing we should remember is that // hours are given in the 24 hour format // that means 8pm will mean 20 as hours // we can also use a short hand way // and combine the new date object creation // with the get hours method let timeOfDay = new Date().getHours(); if (timeOfDay >= 20) { console.log("Turning on Dark Mode..."); } else { console.log("Do not turn on the Dark Mode"); } // since current time is over 8pm, // in this case we expect to get turn on Dark Mode. // which is also the reuslt we get as we can see from // the console output.
上記のコードを実行すると、次のコンソール ログが得られます。
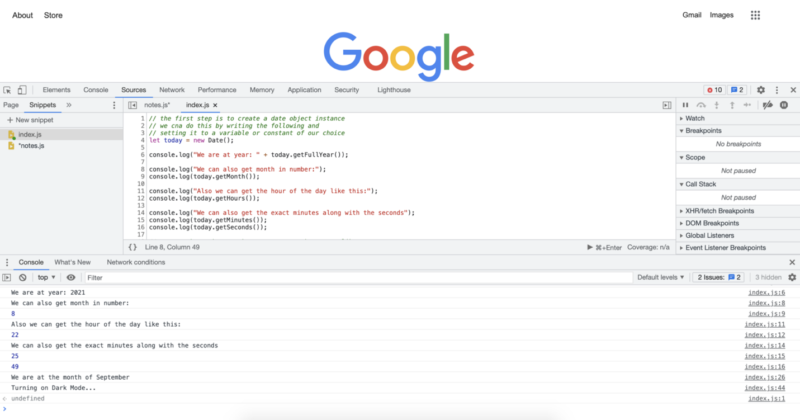
Map() メソッド
map メソッドは、多くのコード行を節約できる非常に便利なメソッドであり、使い方によってはコードをよりきれいにすることができます。 配列をループするために使用する場合、基本的に for ループの使用を置き換えます。 map() メソッドの例をいくつか示します。
// lets create an array we will use for mapping let someNumbers = [1, 2, 3, 4, 5]; // lets also create the functions we will // provide to the map method function doubleNums(num1){ return num1 * 2; } function squareNums(num1){ return num1 * num1; } function add100(num1){ return num1 + 100; } console.log("Doubled numbers array:"); console.log(someNumbers.map(doubleNums)); console.log("Squared numbers array:"); console.log(someNumbers.map(squareNums)); console.log("100 added to each of the element in the umbers array:"); console.log(someNumbers.map(add100)); // map() method will loop over each of the // items in a given array and apply the // provided function // note that we do not include paranthesis // after the function names, this would call the function // instead we pass the function name, // and map() method calls them when it needs to
上記のコードを実行すると、次のコンソール ログが得られます。

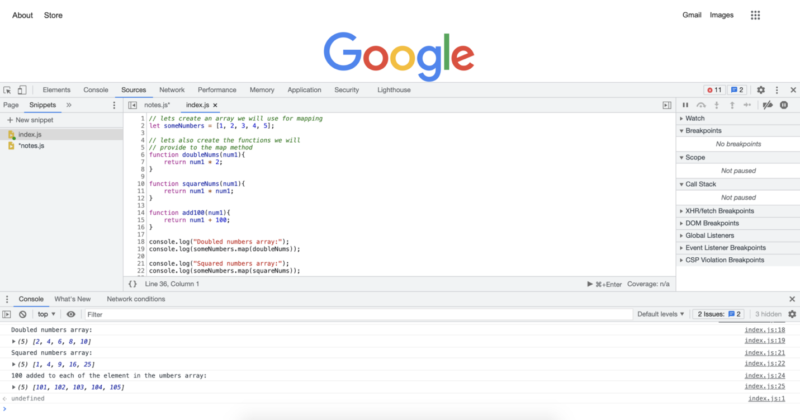
Filter() メソッド
filter() メソッドと map() メソッドは、どちらもかなり一般的な JavaScript メソッドです。 これらは、先ほど見た map() メソッドに非常に似ています。 map() メソッドを使用すると、任意の関数を渡すことができ、その関数は配列内の各要素に適用されます。 filter() メソッドを使用してフィルター条件を渡すと、フィルター メソッドは配列内のすべてのアイテムをループし、基準を通過したアイテムのみが残る新しい配列を返します。 その例をいくつか見てみましょう。
// lets first create an array // to apply the filter() method let someNumbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21]; function checkEven(num1){ if (num1 % 2 == 0){ return num1; } } function checkOdd(num1){ if (num1 % 2 == 1){ return num1; } } function over13(num1){ if (num1 > 13){ return num1; } } function divisibleByFive(num){ if (num % 5 == 0){ return num; } } console.log("Even numbers from the list:"); console.log(someNumbers.filter(checkEven)); console.log("Odd numbers from the list:"); console.log(someNumbers.filter(checkOdd)); console.log("Numbers over 13 from the array:"); console.log(someNumbers.filter(over13)); console.log("Numbers divisible by 5 from the array:"); console.log(someNumbers.filter(divisibleByFive));上記のコードを実行すると、次のコンソール ログが得られます。
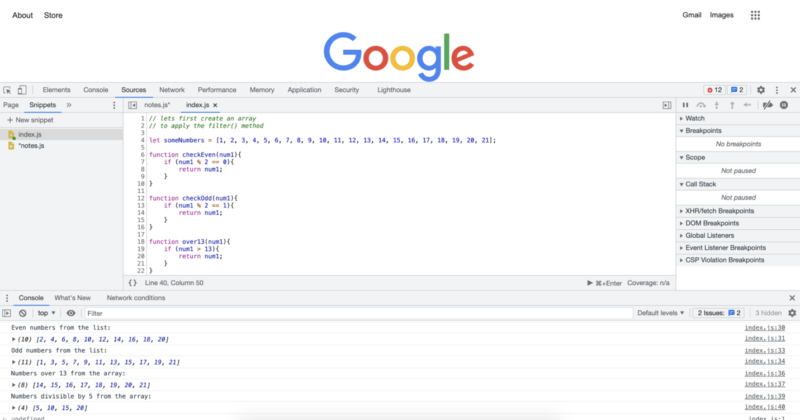
アロー機能
関数は JavaScript で非常に一般的であり、パフォーマンスを向上させたり、コードをクリーンにするために多くの最適化が行われていると言ったことを覚えていますか? アロー関数もその一つです。 アロー関数は、ファット アローと呼ばれることもあります。 それらは基本的に、関数を記述するためのはるかに短い方法を提供します。 これらは、先ほど見た JavaScript メソッドでも非常に一般的に使用されます。 いくつかの例でそれらを見てみましょう:
// JavaScript provides multiple levels of // code shortening with arrow functions depending on your exact code // Essantially the longest way we can write a function is // the way we always write them without using the arrow functions // lets start with an array to apply the arrow functions let someNumbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21]; console.log("Original Array:"); console.log(someNumbers); // in the previous examples we have applied many functions // after creating them as regular named functions // In this example we will apply the exact transformations // so that you can see the both extremes in code lenght // double every number in the array: console.log("Double every number in the array:") console.log(someNumbers.map(num => num * 2)); // square every number in the array: console.log("Square every number in the array:") console.log(someNumbers.map(num => num * num)); // add 100 to every number in the array: console.log("Add 100 to every number in the array:") console.log(someNumbers.map(num => num + 100)); // Only keep the even numbers in the array: console.log("Only keep the even numbers in the array:") console.log(someNumbers.filter(num => num % 2 == 0)); // Only keep the odd numbers in the array: console.log("Only keep the odd numbers in the array:") console.log(someNumbers.filter(num => num % 2 == 1)); // Only keep the numbers that are evenly divisible by 5: console.log("Only keep the numbers that are evenly divisible by 5:") console.log(someNumbers.filter(num => num % 5 == 0)); // Only keep the numbers that are over 13: console.log("Only keep the numbers that are over 13:") console.log(someNumbers.filter(num => num > 13));
上記のコードを実行すると、次のコンソール ログが得られます。
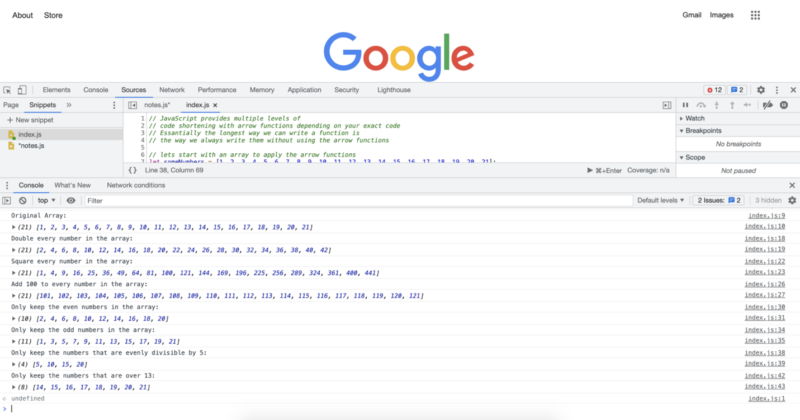
次のチュートリアルでは、これまで見てきたことの概要を説明し、次に何が起こるかを見ていきます。
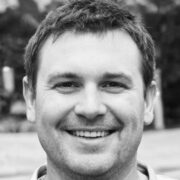
著者: ロバート・ホイットニー
JavaScript のエキスパートであり、IT 部門を指導するインストラクター。 彼の主な目標は、コーディング中に効果的に協力する方法を他の人に教えることで、チームの生産性を向上させることです。
10 のブログ投稿で初心者から上級者までの JavaScript コース:
- JavaScript でコーディングを開始するには?
- JavaScript の基本
- JavaScript の変数とさまざまなデータ型
- スニペットと制御構造
- while ループと for ループ
- Java 配列
- JavaScript 関数
- JavaScript オブジェクト
- JavaScript メソッドなど
- JavaScriptコースのまとめ