Métodos JavaScript e muito mais. Parte 9 Curso de JavaScript do iniciante ao avançado em 10 postagens no blog
Publicados: 2021-11-09Esta é a parte 9 da série de postagens do blog JavaScript que o levará do iniciante ao avançado. Desta vez vamos explorar os seguintes tópicos: métodos JavaScript, valores de parâmetros padrão, o objeto Date em JavaScript e a função de seta. Ao final desta série, você conhecerá todos os conceitos básicos necessários para começar a codificar em JavaScript. Se você não leu a postagem anterior do blog sobre objetos JavaScript, pode fazê-lo aqui. Sem mais delongas, vamos começar com o nono tutorial.
Métodos JavaScript e mais – índice:
- Valores de parâmetro padrão
- O objeto Date em JavaScript
- Método Map()
- Método Filter()
- Função de seta
Até agora vimos muitos conceitos e tópicos em JavaScript, mas ainda existem alguns comumente usados que não descobrimos. Neste tutorial, veremos do que se trata. O primeiro são os valores de parâmetro padrão em JavaScript.
Valores de parâmetro padrão
As funções são usadas com extrema frequência na programação, e quando algo é usado com frequência, não há apenas frameworks como React que aproveitam funções JavaScript, mas há otimizações ainda mais desenvolvidas para obter ainda mais das funções JavaScript. Um dos principais recursos que temos em funções é chamado de valores de parâmetro padrão. Os parâmetros padrão nos permitem escrever códigos mais seguros que podem fazer suposições seguras sobre a entrada do usuário. Isso também beneficia o usuário, fornecendo uma configuração padrão que pode facilitar a escolha de suas opções. Vejamos alguns exemplos disso.
// assume you are developing a website for a coffee shop // and most people want to get a medium size americano // knowing this you want to make it easir for people to // order their coffee with less hustle function orderCoffee(typeOfCoffee="americano", sizeOfCoffee="medium", numberOfCoffee=1){ return (numberOfCoffee + " " + sizeOfCoffee + " size " + typeOfCoffee + " is being prepared. "); } console.log("Default order:"); console.log(orderCoffee()); // this way, when an average customer orders online, // it will be much easier for them to order their daily coffee // so much so that they will be able to order it with a single button // alternatively people can also customize their coffee // by changing the inputs console.log("Custom order:"); console.log(orderCoffee("latte", "large", 2)); console.log("Another custom order:"); console.log(orderCoffee("macchiato", "small", 1)); // it is also possible to change only part of the inputs // and leverage the default parameters // for the rest of the input fields console.log("Partially customized order:"); console.log(orderCoffee("iced coffee"));
A execução do código acima nos dá a seguinte saída:
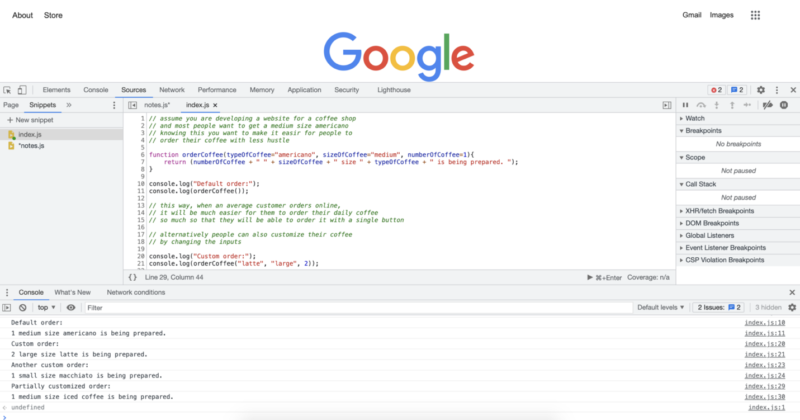
O objeto Date em JavaScript
O objeto Date em JavaScript é bastante usado, especialmente no desenvolvimento web. Podemos usar o objeto Date para executar funções sensíveis ao tempo, como alterar as configurações de exibição para modo escuro, modo claro ou qualquer outro modo que o usuário possa preferir. Também podemos usar as informações de data conforme necessário dentro do projeto em que estamos trabalhando. Aqui estão alguns exemplos do objeto Date em ação:
// the first step is to create a date object instance // we cna do this by writing the following and // setting it to a variable or constant of our choice let today = new Date(); console.log("We are at year: " + today.getFullYear()); console.log("We can also get month in number:"); console.log(today.getMonth()); console.log("Also we can get the hour of the day like this:"); console.log(today.getHours()); console.log("We can also get the exact minutes along with the seconds"); console.log(today.getMinutes()); console.log(today.getSeconds()); // once we have these numbers we can use them as we like // if we want we can display them or make decisions based on them. // if we want to display month with a name // rather than a number, we can also achieve that // with the following const months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]; console.log("We are at the month of " + months[today.getMonth()]); // what we just did was to create an array to store month names // and then choose the correct month using an index value // provided by the .getMonth() method. // if we wanted to turn on dark mode after 8 pm, // we could do that with the following code // one of the first thing we should remember is that // hours are given in the 24 hour format // that means 8pm will mean 20 as hours // we can also use a short hand way // and combine the new date object creation // with the get hours method let timeOfDay = new Date().getHours(); if (timeOfDay >= 20) { console.log("Turning on Dark Mode..."); } else { console.log("Do not turn on the Dark Mode"); } // since current time is over 8pm, // in this case we expect to get turn on Dark Mode. // which is also the reuslt we get as we can see from // the console output.
A execução do código acima nos dará os seguintes logs do console:
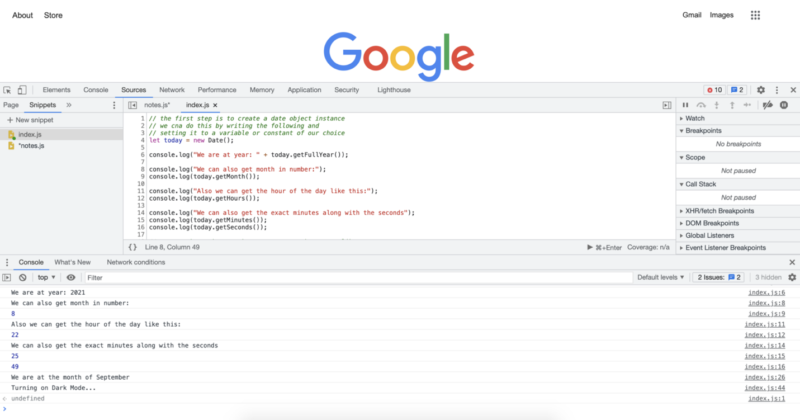
Método Map()
O método map é um método muito útil que pode economizar muitas linhas de código e, dependendo de como você o usa, pode tornar seu código muito mais limpo. Essencialmente, ele substitui o uso de um loop for quando você o usa para fazer um loop em uma matriz. Aqui estão alguns exemplos do método map().
// lets create an array we will use for mapping let someNumbers = [1, 2, 3, 4, 5]; // lets also create the functions we will // provide to the map method function doubleNums(num1){ return num1 * 2; } function squareNums(num1){ return num1 * num1; } function add100(num1){ return num1 + 100; } console.log("Doubled numbers array:"); console.log(someNumbers.map(doubleNums)); console.log("Squared numbers array:"); console.log(someNumbers.map(squareNums)); console.log("100 added to each of the element in the umbers array:"); console.log(someNumbers.map(add100)); // map() method will loop over each of the // items in a given array and apply the // provided function // note that we do not include paranthesis // after the function names, this would call the function // instead we pass the function name, // and map() method calls them when it needs to
A execução do código acima nos dará os seguintes logs do console:

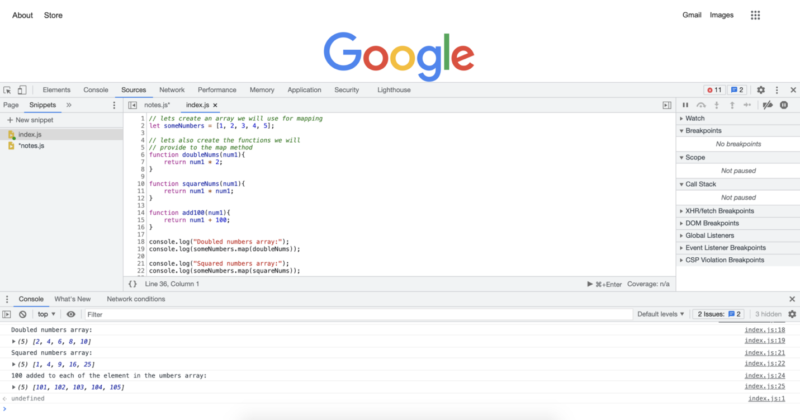
Método Filter()
O método filter(), junto com o método map(), são métodos JavaScript bastante comuns. Eles são muito semelhantes ao método map() que acabamos de ver. Com o método map() podemos passar qualquer função, e essa função está sendo aplicada a cada um dos elementos de um array. Com o método filter(), passaremos um critério de filtro e o método de filtro fará um loop sobre todos os itens em um array e retornará um novo array com apenas os itens que passam nos critérios que permanecerão. Vejamos alguns exemplos disso:
// lets first create an array // to apply the filter() method let someNumbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21]; function checkEven(num1){ if (num1 % 2 == 0){ return num1; } } function checkOdd(num1){ if (num1 % 2 == 1){ return num1; } } function over13(num1){ if (num1 > 13){ return num1; } } function divisibleByFive(num){ if (num % 5 == 0){ return num; } } console.log("Even numbers from the list:"); console.log(someNumbers.filter(checkEven)); console.log("Odd numbers from the list:"); console.log(someNumbers.filter(checkOdd)); console.log("Numbers over 13 from the array:"); console.log(someNumbers.filter(over13)); console.log("Numbers divisible by 5 from the array:"); console.log(someNumbers.filter(divisibleByFive));A execução do código acima nos dará os seguintes logs do console:
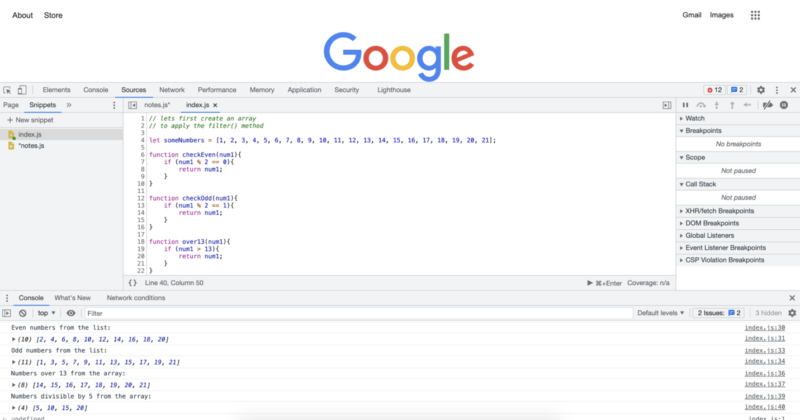
Função de seta
Lembra quando dissemos que as funções são extremamente comuns em JavaScript e que muitas otimizações são feitas para obter um código ainda mais performático ou limpo? Bem, as funções de seta são uma delas. As funções de seta às vezes também são chamadas de seta gorda. Eles essencialmente fornecem uma maneira muito mais curta de escrever suas funções. Eles também são muito usados com os métodos JavaScript que acabamos de ver. Vamos vê-los com alguns exemplos:
// JavaScript provides multiple levels of // code shortening with arrow functions depending on your exact code // Essantially the longest way we can write a function is // the way we always write them without using the arrow functions // lets start with an array to apply the arrow functions let someNumbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21]; console.log("Original Array:"); console.log(someNumbers); // in the previous examples we have applied many functions // after creating them as regular named functions // In this example we will apply the exact transformations // so that you can see the both extremes in code lenght // double every number in the array: console.log("Double every number in the array:") console.log(someNumbers.map(num => num * 2)); // square every number in the array: console.log("Square every number in the array:") console.log(someNumbers.map(num => num * num)); // add 100 to every number in the array: console.log("Add 100 to every number in the array:") console.log(someNumbers.map(num => num + 100)); // Only keep the even numbers in the array: console.log("Only keep the even numbers in the array:") console.log(someNumbers.filter(num => num % 2 == 0)); // Only keep the odd numbers in the array: console.log("Only keep the odd numbers in the array:") console.log(someNumbers.filter(num => num % 2 == 1)); // Only keep the numbers that are evenly divisible by 5: console.log("Only keep the numbers that are evenly divisible by 5:") console.log(someNumbers.filter(num => num % 5 == 0)); // Only keep the numbers that are over 13: console.log("Only keep the numbers that are over 13:") console.log(someNumbers.filter(num => num > 13));
A execução do código acima nos dará os seguintes logs do console:
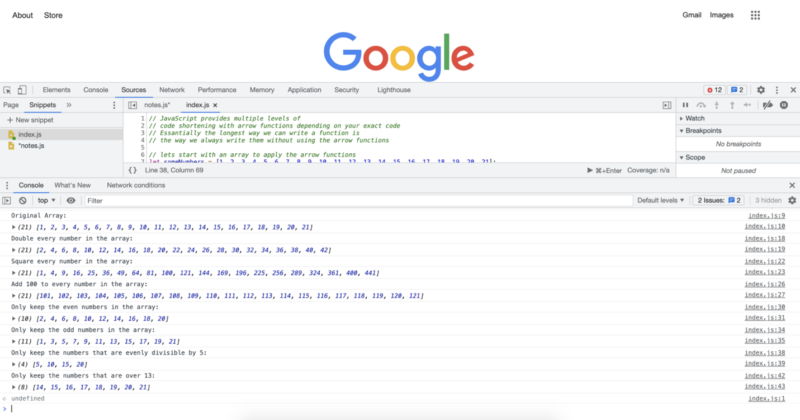
No próximo tutorial teremos uma visão geral do que vimos e veremos o que vem a seguir.
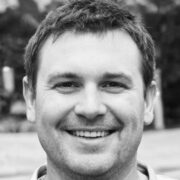
Autor: Robert Whitney
Especialista e instrutor em JavaScript que treina departamentos de TI. Seu principal objetivo é aumentar a produtividade da equipe, ensinando outras pessoas a cooperar efetivamente durante a codificação.
Curso de JavaScript do iniciante ao avançado em 10 posts:
- Como começar a codificar em JavaScript?
- Noções básicas de JavaScript
- Variáveis e diferentes tipos de dados em JavaScript
- Trechos e estruturas de controle
- loops while e loops for
- Matriz Java
- Funções JavaScript
- Objetos JavaScript
- Métodos JavaScript e muito mais
- Resumo do curso de JavaScript