Методы JavaScript и многое другое. Часть 9 Курс JavaScript от начального до продвинутого в 10 сообщениях в блоге
Опубликовано: 2021-11-09Это девятая часть серии сообщений в блоге о JavaScript, которая проведет вас от новичка до продвинутого уровня. На этот раз мы рассмотрим следующие темы: методы JavaScript, значения параметров по умолчанию, объект Date в JavaScript и функция стрелки. К концу этой серии вы будете знать все основы, которые вам нужно знать, чтобы начать программировать на JavaScript. Если вы не читали предыдущую запись в блоге об объектах JavaScript, вы можете сделать это здесь. Без лишних слов, давайте начнем с девятого урока.
Методы JavaScript и многое другое — оглавление:
- Значения параметров по умолчанию
- Объект Date в JavaScript
- Метод карты()
- Метод Фильтр()
- Функция стрелки
До сих пор мы видели много концепций и тем в JavaScript, но все еще есть некоторые часто используемые, которые мы не обнаружили. В этом уроке мы увидим, что они из себя представляют. Первый — это значения параметров по умолчанию в JavaScript.
Значения параметров по умолчанию
Функции очень часто используются в программировании, и когда что-то используется так часто, существуют не только такие фреймворки, как React, которые используют функции JavaScript, но и разработаны дополнительные оптимизации, чтобы получить больше от функций JavaScript. Одна из основных функций, которые мы имеем в функциях, называется значениями параметров по умолчанию. Параметры по умолчанию позволяют нам писать более безопасные коды, которые могут делать безопасные предположения о пользовательском вводе. Это также приносит пользу пользователю, предоставляя ему настройку по умолчанию, которая также может упростить выбор из их вариантов. Давайте посмотрим на некоторые примеры.
// assume you are developing a website for a coffee shop // and most people want to get a medium size americano // knowing this you want to make it easir for people to // order their coffee with less hustle function orderCoffee(typeOfCoffee="americano", sizeOfCoffee="medium", numberOfCoffee=1){ return (numberOfCoffee + " " + sizeOfCoffee + " size " + typeOfCoffee + " is being prepared. "); } console.log("Default order:"); console.log(orderCoffee()); // this way, when an average customer orders online, // it will be much easier for them to order their daily coffee // so much so that they will be able to order it with a single button // alternatively people can also customize their coffee // by changing the inputs console.log("Custom order:"); console.log(orderCoffee("latte", "large", 2)); console.log("Another custom order:"); console.log(orderCoffee("macchiato", "small", 1)); // it is also possible to change only part of the inputs // and leverage the default parameters // for the rest of the input fields console.log("Partially customized order:"); console.log(orderCoffee("iced coffee"));
Выполнение приведенного выше кода дает нам следующий результат:
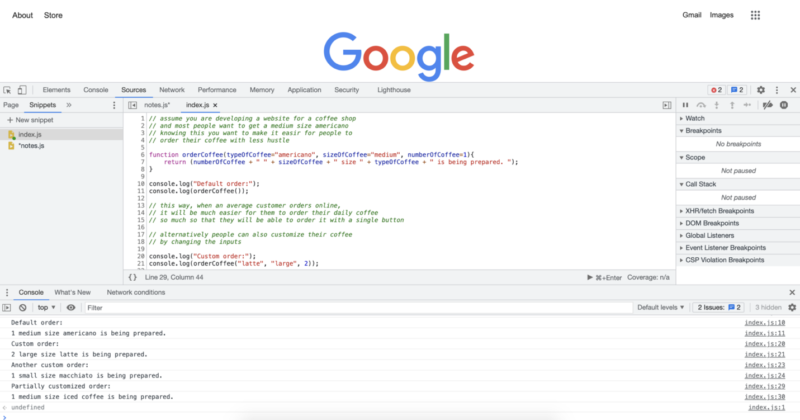
Объект Date в JavaScript
Объект Date в JavaScript используется довольно часто, особенно в веб-разработке. Мы можем использовать объект Date для выполнения чувствительных ко времени функций, таких как изменение настроек дисплея в темный режим, светлый режим или любой другой режим, который может предпочесть пользователь. Мы также можем использовать информацию о дате по мере необходимости внутри проекта, над которым мы работаем. Вот несколько примеров объекта Date в действии:
// the first step is to create a date object instance // we cna do this by writing the following and // setting it to a variable or constant of our choice let today = new Date(); console.log("We are at year: " + today.getFullYear()); console.log("We can also get month in number:"); console.log(today.getMonth()); console.log("Also we can get the hour of the day like this:"); console.log(today.getHours()); console.log("We can also get the exact minutes along with the seconds"); console.log(today.getMinutes()); console.log(today.getSeconds()); // once we have these numbers we can use them as we like // if we want we can display them or make decisions based on them. // if we want to display month with a name // rather than a number, we can also achieve that // with the following const months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]; console.log("We are at the month of " + months[today.getMonth()]); // what we just did was to create an array to store month names // and then choose the correct month using an index value // provided by the .getMonth() method. // if we wanted to turn on dark mode after 8 pm, // we could do that with the following code // one of the first thing we should remember is that // hours are given in the 24 hour format // that means 8pm will mean 20 as hours // we can also use a short hand way // and combine the new date object creation // with the get hours method let timeOfDay = new Date().getHours(); if (timeOfDay >= 20) { console.log("Turning on Dark Mode..."); } else { console.log("Do not turn on the Dark Mode"); } // since current time is over 8pm, // in this case we expect to get turn on Dark Mode. // which is also the reuslt we get as we can see from // the console output.
Выполнение приведенного выше кода даст нам следующие журналы консоли:
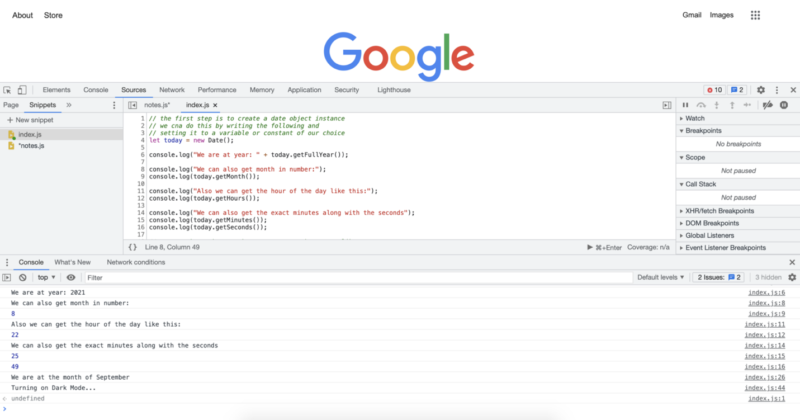
Метод карты()
Метод карты — очень полезный метод, который может сэкономить вам много строк кода и, в зависимости от того, как вы его используете, может сделать ваш код намного чище. По сути, он заменяет использование цикла for, когда вы используете его для перебора массива. Вот несколько примеров метода map().
// lets create an array we will use for mapping let someNumbers = [1, 2, 3, 4, 5]; // lets also create the functions we will // provide to the map method function doubleNums(num1){ return num1 * 2; } function squareNums(num1){ return num1 * num1; } function add100(num1){ return num1 + 100; } console.log("Doubled numbers array:"); console.log(someNumbers.map(doubleNums)); console.log("Squared numbers array:"); console.log(someNumbers.map(squareNums)); console.log("100 added to each of the element in the umbers array:"); console.log(someNumbers.map(add100)); // map() method will loop over each of the // items in a given array and apply the // provided function // note that we do not include paranthesis // after the function names, this would call the function // instead we pass the function name, // and map() method calls them when it needs to
Выполнение приведенного выше кода даст нам следующие журналы консоли:

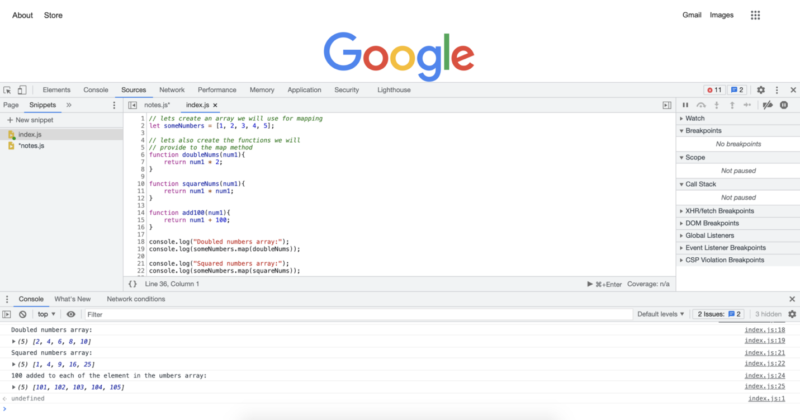
Метод Фильтр()
Метод filter() вместе с методом map() являются довольно распространенными методами JavaScript. Они очень похожи на метод map(), который мы только что видели. С помощью метода map() мы можем передать любую функцию, и эта функция применяется к каждому элементу массива. С помощью метода filter() мы передадим критерий фильтра, и метод фильтра будет перебирать все элементы в массиве и будет возвращать новый массив, в котором останутся только элементы, соответствующие критериям. Давайте посмотрим на несколько примеров:
// lets first create an array // to apply the filter() method let someNumbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21]; function checkEven(num1){ if (num1 % 2 == 0){ return num1; } } function checkOdd(num1){ if (num1 % 2 == 1){ return num1; } } function over13(num1){ if (num1 > 13){ return num1; } } function divisibleByFive(num){ if (num % 5 == 0){ return num; } } console.log("Even numbers from the list:"); console.log(someNumbers.filter(checkEven)); console.log("Odd numbers from the list:"); console.log(someNumbers.filter(checkOdd)); console.log("Numbers over 13 from the array:"); console.log(someNumbers.filter(over13)); console.log("Numbers divisible by 5 from the array:"); console.log(someNumbers.filter(divisibleByFive));Выполнение приведенного выше кода даст нам следующие журналы консоли:
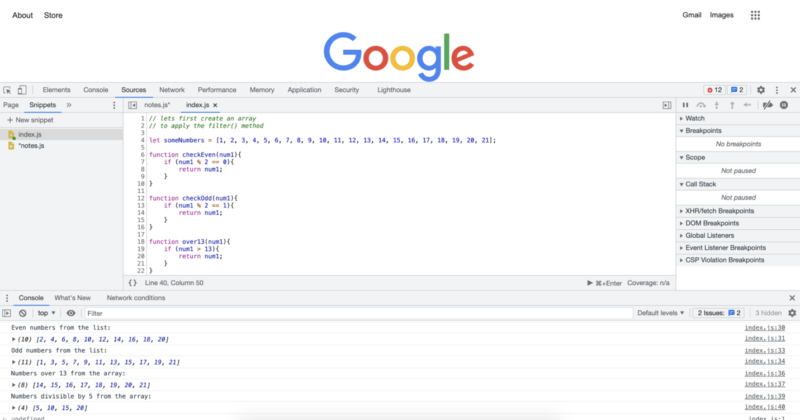
Функция стрелки
Помните, мы говорили, что функции чрезвычайно распространены в JavaScript, и для них сделано много оптимизаций, чтобы получить более производительный или чистый код? Что ж, стрелочные функции — одна из них. Стрелочные функции иногда также называют жирной стрелкой. По сути, они обеспечивают гораздо более короткий способ написания ваших функций. Они также очень часто используются с методами JavaScript, которые мы только что видели. Давайте рассмотрим их на нескольких примерах:
// JavaScript provides multiple levels of // code shortening with arrow functions depending on your exact code // Essantially the longest way we can write a function is // the way we always write them without using the arrow functions // lets start with an array to apply the arrow functions let someNumbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21]; console.log("Original Array:"); console.log(someNumbers); // in the previous examples we have applied many functions // after creating them as regular named functions // In this example we will apply the exact transformations // so that you can see the both extremes in code lenght // double every number in the array: console.log("Double every number in the array:") console.log(someNumbers.map(num => num * 2)); // square every number in the array: console.log("Square every number in the array:") console.log(someNumbers.map(num => num * num)); // add 100 to every number in the array: console.log("Add 100 to every number in the array:") console.log(someNumbers.map(num => num + 100)); // Only keep the even numbers in the array: console.log("Only keep the even numbers in the array:") console.log(someNumbers.filter(num => num % 2 == 0)); // Only keep the odd numbers in the array: console.log("Only keep the odd numbers in the array:") console.log(someNumbers.filter(num => num % 2 == 1)); // Only keep the numbers that are evenly divisible by 5: console.log("Only keep the numbers that are evenly divisible by 5:") console.log(someNumbers.filter(num => num % 5 == 0)); // Only keep the numbers that are over 13: console.log("Only keep the numbers that are over 13:") console.log(someNumbers.filter(num => num > 13));
Выполнение приведенного выше кода даст нам следующие журналы консоли:
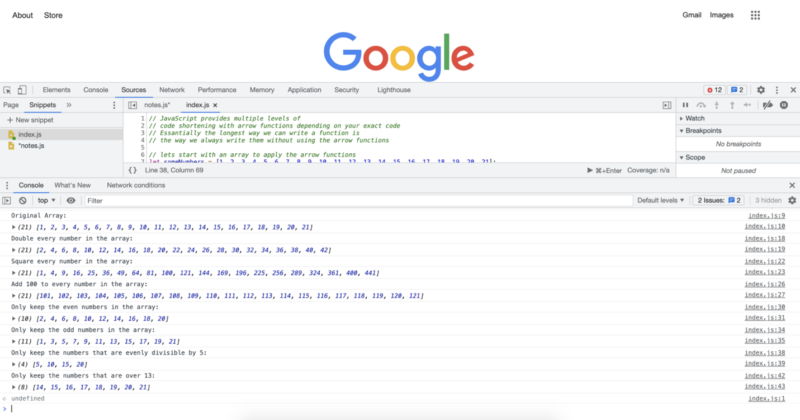
В следующем уроке у нас будет обзор того, что мы видели, и посмотрим, что будет дальше.
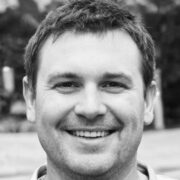
Автор: Роберт Уитни
Эксперт и инструктор по JavaScript, который тренирует ИТ-отделы. Его главная цель — повысить продуктивность команды, научив других эффективно сотрудничать при написании кода.
Курс JavaScript от начального до продвинутого в 10 сообщениях в блоге:
- Как начать программировать на JavaScript?
- Основы JavaScript
- Переменные и разные типы данных в JavaScript
- Фрагменты и управляющие структуры
- Циклы while и циклы for
- Массив Java
- Функции JavaScript
- Объекты JavaScript
- Методы JavaScript и многое другое
- Краткое содержание курса JavaScript