การใช้งาน Python ในทางปฏิบัติ Part 11 Python Course ตั้งแต่ Beginner ถึง Advanced ใน 11 บล็อกโพสต์
เผยแพร่แล้ว: 2022-01-27ในบทความนี้จะช่วยให้ผู้อ่านใช้การเรียนรู้จากบล็อกก่อนหน้านี้ทั้งหมดเพื่อสร้างโครงการขนาดเล็ก คุณจะค้นพบแอปพลิเคชัน Python ในทางปฏิบัติ เราจะใช้ Visual Studio Code เป็นตัวแก้ไขโค้ดของเรา หากคุณยังไม่ได้ติดตั้ง Visual Studio Code คำแนะนำจะมีให้ในบล็อกแรก
แอปพลิเคชั่น Python ในทางปฏิบัติ - สร้างเกมเดาตัวเลข
โปรเจ็กต์ขนาดเล็กนี้จะน่าตื่นเต้นที่ได้เรียนรู้วิธีการใช้ฟังก์ชันและสิ่งอื่น ๆ ส่วนใหญ่ที่เราได้เรียนรู้ในบล็อกที่แล้ว เกมมินิโปรเจ็กต์นี้สร้างตัวเลขสุ่มตั้งแต่ 1 ถึง 1,000 หรือหากคุณต้องการให้ง่าย คุณสามารถลดช่วงและผู้ใช้ที่เล่นเกมจะต้องเดาตัวเลข ฟังดูน่าตื่นเต้นใช่ไหม สิ่งที่ทำให้น่าตื่นเต้นมากขึ้นคือเราสามารถให้ตัวชี้นำแก่ผู้ใช้ได้หากเขาเดาตัวเลขผิดเพื่อให้พวกเขาสามารถเดาตัวเลขได้อย่างถูกต้อง
มาสร้างพิมพ์เขียวสำหรับเกมด้วยแอพพลิเคชั่น Python กัน
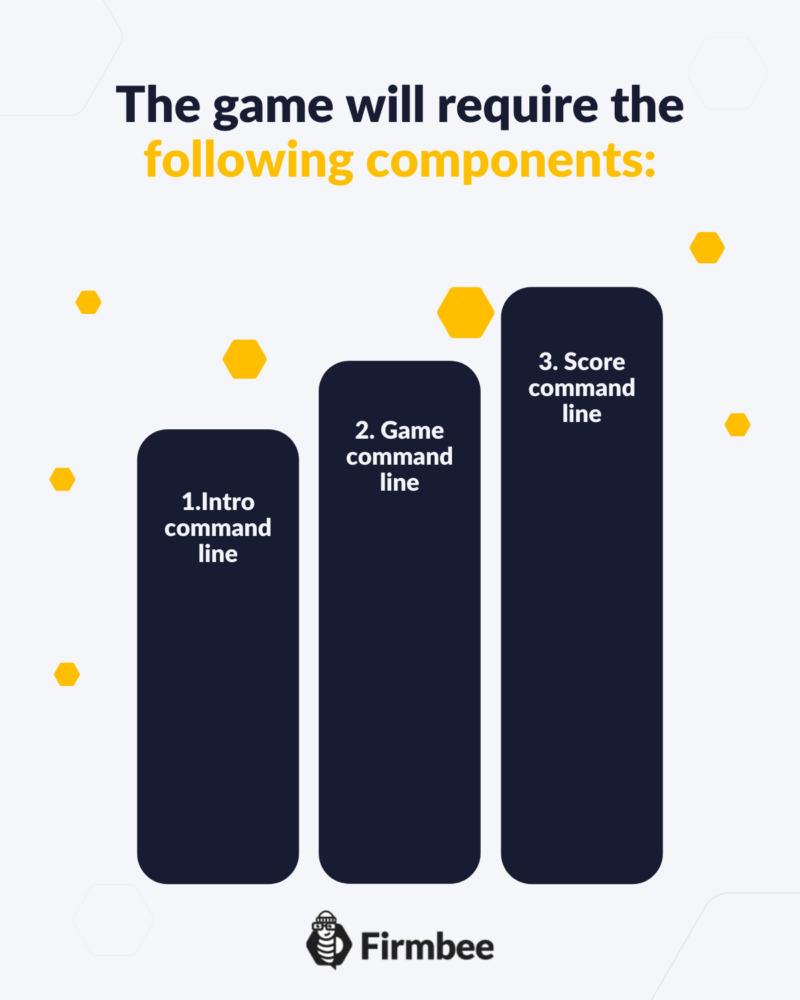
บทนำบรรทัดคำสั่ง
ในบรรทัดคำสั่ง intro เราจะขอให้ผู้ใช้เดาตัวเลข เราจะถามชื่อและอายุของเขา จากนั้นเราจะถามเขาว่าเขาต้องการเล่นเกมหรือไม่ ลองทำสิ่งนี้ในรหัส
# Intro Panel Command line print("Welcome to the guessnum") name=input("what is your name?") print(f"Hello {name}")
Output: Welcome to the guessnum Hello john
ดังที่เห็นได้ชัดเจนว่าเราแนะนำเกมของเราให้กับผู้ใช้ก่อนแล้วจึงถามชื่อผู้ใช้ของพวกเขา เราทักทายพวกเขาโดยใช้ชื่อที่บันทึกไว้ ทีนี้ลองถามอายุผู้ใช้ดู
# Intro Panel Command line print("Welcome to the guessnum") name=input("what is your name?") age=int(input(f"Hello {name}, what is your age?")) print(f"Hello {name}")
Output: Welcome to the guessnum Hello john
ในที่นี้ เราจะเห็น fstring ซึ่งเป็นอีกทางเลือกหนึ่งของการจัดรูปแบบ หากเราเขียน f ตามด้วยสตริง เราสามารถใช้ตัวแปรที่เก็บไว้ภายใน “{}” ได้โดยตรง
ตอนนี้เราสามารถเห็นแผงแนะนำส่วนใหญ่ได้แล้ว ทีนี้ลองถามผู้ใช้ว่าอยากเล่นเกมไหม และถ้าอยากเล่นเกม ให้ขอให้เขาเดาตัวเลข แล้วเราบอกได้เลยว่าถูกหรือไม่ แต่ก่อนให้ผู้ใช้เดาเลขเราต้องเตรียมเลขโปรแกรมไว้ให้พร้อม เรามาดูกันว่ามันทำอย่างไรในรหัส
# Intro Panel Command line print("Welcome to the guessnum") name=input("what is your name?") age=int(input(f"Hello {name}, what is your age?")) choice=input(f"Hello {name}, would you like to play the game? y/n") if choice=="y": pass else: print("exiting") exit
ตอนนี้ เรากำลังสร้างข้อความแจ้งอีกครั้งซึ่งจะถามผู้ใช้ว่าเขาต้องการเล่นเกมหรือไม่ และเราจะใช้เงื่อนไขที่เราได้เรียนรู้ในบล็อกก่อนหน้านี้เพื่อดำเนินการต่อ ถ้าเขาตอบว่าใช่ และถ้าไม่ใช่ ให้ออกจากเกม ตอนนี้เรามาขยายเกมของเราต่อไปและขอหมายเลขจากผู้ใช้ แต่ก่อนหน้านั้นมาทำให้รหัสของเราเลือกหมายเลขสุ่ม
# Intro Panel Command line import random print("Welcome to the guessnum") name=input("what is your name?") age=int(input(f"Hello {name}, what is your age?")) choice=input(f"Hello {name}, would you like to play the game? y/n") if choice=="y": number=int(random.randint(1,5)) guess=int(input("Please input your guess")) print(f"your guess is {guess}") else: print("exiting") exit
Output: Welcome to the guessnum your guess is 2
ตอนนี้เราได้เพิ่มการนำเข้าที่เรียกว่าการสุ่ม ซึ่งเลือกตัวเลขสุ่มจากช่วงที่กำหนด ฟังก์ชั่นเป็น random.randint (เริ่ม, สิ้นสุด) จากนั้นเรากำลังขอให้ผู้ใช้ของเราเดาตัวเลขและเรากำลังพิมพ์ผู้ใช้ของเราเดา
มาพิมพ์การเดาของโปรแกรมของเราด้วย
# Intro Panel Command line import random print("Welcome to the guessnum") name=input("what is your name?") age=int(input(f"Hello {name}, what is your age?")) choice=input(f"Hello {name}, would you like to play the game? y/n") if choice=="y": number=int(random.randint(1,5)) guess=int(input("Please input your guess")) print(f"your guess is {guess} and program's guess is {number}") else: print("exiting") exit
output: Welcome to the guessnum your guess is 2 and the program's guess is 5
ดังนั้นเราจะเห็นได้ว่าเรามาเกือบครึ่งทางแล้ว เรามีการเดาโปรแกรมและการเดาของผู้ใช้ ตอนนี้เราสามารถเปรียบเทียบและพิมพ์ได้ว่าผู้ใช้ถูกต้องหรือไม่
# Intro Panel Command line import random print("Welcome to the guessnum") name=input("what is your name?") age=int(input(f"Hello {name}, what is your age?")) choice=input(f"Hello {name}, would you like to play the game? y/n") if choice=="y": number=int(random.randint(1,5)) guess=int(input("Please input your guess")) if guess==number: print("you guessed it right!!!") print(f"your guess is {guess} and program's guess is {number}. Sorry!!! your guess is wrong") else: print("exiting") exit
output: Welcome to the guessnum your guess is 2 and the program's guess is 1. Sorry!!! your guess is wrong
อย่างที่คุณเห็น ฉันเดาผิด บางทีคุณอาจเดาถูกก็ได้ เกมนี้สามารถทำให้น่าสนใจยิ่งขึ้นโดยการเพิ่มปัจจัยคะแนน ตอนนี้ มาโค้ดสำหรับปัจจัยคะแนนกัน

# Intro Panel Command line import random print("Welcome to the guessnum") name=input("what is your name?") age=int(input(f"Hello {name}, what is your age?")) choice=input(f"Hello {name}, would you like to play the game? y/n") correct=0 while(choice=="y"): number=int(random.randint(1,5)) guess=int(input("Please input your guess")) if guess==number: print("you guessed it right!!!") correct+=1 choice=input(f"Hello {name}, would you like to continue the game? y/n") print(f"your guess is {guess} and program's guess is {number}. Sorry!!! your guess is wrong") choice=input(f"Hello {name}, would you like to continue the game? y/n") else: print(f"your score is {correct}") print("exiting") exit
output: Welcome to the guessnum your guess is 1 and program's guess is 5. Sorry!!! your guess is wrong your guess is 2 and program's guess is 3. Sorry!!! your guess is wrong your guess is 3 and program's guess is 2. Sorry!!! your guess is wrong your guess is 4 and program's guess is 3. Sorry!!! your guess is wrong your guess is 1 and program's guess is 2. Sorry!!! your guess is wrong your guess is 2 and program's guess is 5. Sorry!!! your guess is wrong your guess is 3 and program's guess is 4. Sorry!!! your guess is wrong your guess is 3 and program's guess is 2. Sorry!!! your guess is wrong your guess is 3 and program's guess is 5. Sorry!!! your guess is wrong your guess is 4 and program's guess is 2. Sorry!!! your guess is wrong your guess is 3 and program's guess is 1. Sorry!!! your guess is wrong your guess is 4 and program's guess is 5. Sorry!!! your guess is wrong your guess is 2 and program's guess is 2. you guessed it right!!! Sorry!!! your guess is wrong your score is 1 exiting
อย่างที่คุณเห็น เราใช้ while loop และเราใช้ตัวแปรใหม่ที่เรียกว่า correct ซึ่งให้คะแนนผู้ใช้แก่เรา ซึ่งเรากำลังพิมพ์งานออกมา
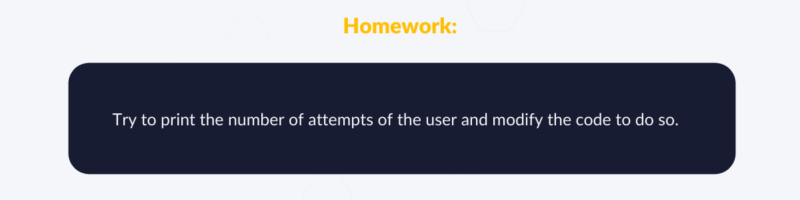
คุณอาจชอบหลักสูตร JavaScript ของเราตั้งแต่ระดับเริ่มต้นไปจนถึงระดับสูง
ยินดีด้วย! ตอนนี้คุณรู้วิธีนำแอปพลิเคชัน Python ไปใช้จริงแล้ว และคุณเรียนจบหลักสูตรอย่างเป็นทางการแล้ว: หลักสูตร Python ตั้งแต่ระดับ Beginner ถึง Advanced ใน 11 บล็อกโพสต์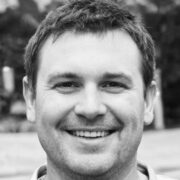
ผู้เขียน: โรเบิร์ต วิทนีย์
ผู้เชี่ยวชาญ JavaScript และผู้สอนที่โค้ชแผนกไอที เป้าหมายหลักของเขาคือการยกระดับประสิทธิภาพการทำงานของทีมโดยการสอนผู้อื่นถึงวิธีการร่วมมืออย่างมีประสิทธิภาพขณะเขียนโค้ด
Python Course จาก Beginner ถึง Advanced ใน 11 บล็อกโพสต์:
- บทนำสู่หลักสูตรไพทอน ส่วนที่ 1 Python Course จาก Beginner ถึง Advanced ใน 11 บล็อกโพสต์
- ตัวแปรและชนิดข้อมูลในภาษาไพทอน ส่วนที่ 2 Python Course จาก Beginner ถึง Advanced ใน 11 บล็อกโพสต์
- Python tuples, รายการ, ชุดและพจนานุกรม ส่วนที่ 3 หลักสูตร Python ตั้งแต่ระดับ Beginner ถึง Advanced ใน 11 บล็อกโพสต์
- ชุด Python และพจนานุกรม ส่วนที่ 4 หลักสูตร Python ตั้งแต่ระดับ Beginner ถึง Advanced ใน 11 บล็อกโพสต์
- คำสั่งเงื่อนไขใน Python ส่วนที่ 5 Python Course จาก Beginner ถึง Advanced ใน 11 บล็อกโพสต์
- ลูปใน Python Part 6 Python Course ตั้งแต่ Beginner ถึง Advanced ใน 11 บล็อกโพสต์
- ฟังก์ชันไพทอน Part 7 Python Course ตั้งแต่ Beginner ถึง Advanced ใน 11 บล็อกโพสต์
- ฟังก์ชันขั้นสูงใน Python Part 8 Python Course ตั้งแต่ Beginner ถึง Advanced ใน 11 บล็อกโพสต์
- คลาส Python และอ็อบเจ็กต์ Part 9 Python Course ตั้งแต่ Beginner ถึง Advanced ใน 11 บล็อกโพสต์
- ไฟล์ในไพทอน Part 10 Python Course ตั้งแต่ Beginner ถึง Advanced ใน 11 บล็อกโพสต์
- การใช้งาน Python ในทางปฏิบัติ Part 11 Python Course ตั้งแต่ Beginner ถึง Advanced ใน 11 บล็อกโพสต์