JavaScript yöntemleri ve daha fazlası. 10 blog gönderisinde Başlangıç Düzeyinden İleri Düzey'e kadar 9. Bölüm JavaScript kursu
Yayınlanan: 2021-11-09Bu, sizi başlangıç seviyesinden ileri seviyeye götürecek JavaScript blog yazısı serisinin 9. bölümüdür. Bu sefer şu konuları inceleyeceğiz: JavaScript yöntemleri, varsayılan parametre değerleri, JavaScript'teki Date nesnesi ve ok işlevi. Bu dizinin sonunda, JavaScript'te kodlamaya başlamak için bilmeniz gereken tüm temel bilgileri öğreneceksiniz. JavaScript nesneleri hakkındaki önceki blog gönderisini okumadıysanız, buradan yapabilirsiniz. Lafı fazla uzatmadan dokuzuncu derse başlayalım.
JavaScript yöntemleri ve daha fazlası – içindekiler tablosu:
- Varsayılan parametre değerleri
- JavaScript'teki Date nesnesi
- Harita() yöntemi
- Filter() Yöntemi
- Ok işlevi
Şimdiye kadar JavaScript'te birçok kavram ve konu gördük, ancak hala yaygın olarak kullanılan ve keşfetmediğimiz bazı kavramlar var. Bu eğitimde, bunların neyle ilgili olduğunu göreceğiz. İlki, JavaScript'teki varsayılan parametre değerleridir.
Varsayılan parametre değerleri
İşlevler programlamada son derece yaygın olarak kullanılır ve bir şey kullanıldığında bu sıklıkla yalnızca JavaScript işlevlerinden yararlanan React gibi çerçeveler değildir, ayrıca JavaScript işlevlerinden daha fazlasını elde etmek için geliştirilmiş daha fazla optimizasyon vardır. Fonksiyonlarda sahip olduğumuz en önemli özelliklerden biri varsayılan parametre değerleridir. Varsayılan parametreler, kullanıcı girişi hakkında güvenli varsayımlar yapabilen daha güvenli kodlar yazmamızı sağlar. Bu aynı zamanda kullanıcıya, seçenekleri arasından seçim yapmayı kolaylaştırabilecek bir varsayılan ayar sağlayarak da fayda sağlar. Buna bazı örnekler görelim.
// assume you are developing a website for a coffee shop // and most people want to get a medium size americano // knowing this you want to make it easir for people to // order their coffee with less hustle function orderCoffee(typeOfCoffee="americano", sizeOfCoffee="medium", numberOfCoffee=1){ return (numberOfCoffee + " " + sizeOfCoffee + " size " + typeOfCoffee + " is being prepared. "); } console.log("Default order:"); console.log(orderCoffee()); // this way, when an average customer orders online, // it will be much easier for them to order their daily coffee // so much so that they will be able to order it with a single button // alternatively people can also customize their coffee // by changing the inputs console.log("Custom order:"); console.log(orderCoffee("latte", "large", 2)); console.log("Another custom order:"); console.log(orderCoffee("macchiato", "small", 1)); // it is also possible to change only part of the inputs // and leverage the default parameters // for the rest of the input fields console.log("Partially customized order:"); console.log(orderCoffee("iced coffee"));
Yukarıdaki kodu çalıştırmak bize aşağıdaki çıktıyı verir:
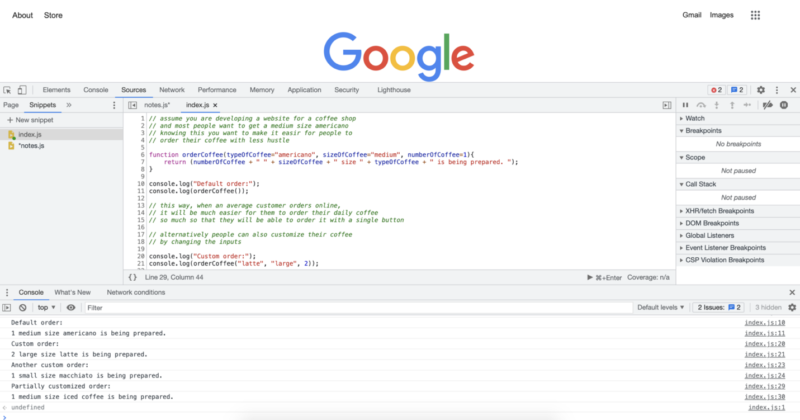
JavaScript'teki Date nesnesi
JavaScript'teki Date nesnesi, özellikle web geliştirmede oldukça yaygın olarak kullanılır. Date nesnesini, ekran ayarlarını karanlık mod, ışık modu veya kullanıcının tercih edebileceği başka herhangi bir mod olarak değiştirmek gibi zamana duyarlı işlevleri gerçekleştirmek için kullanabiliriz. Ayrıca üzerinde çalıştığımız proje içerisinde ihtiyaç duydukça tarih bilgisini de kullanabiliriz. Aşağıda, eylem halindeki Date nesnesinin bazı örnekleri verilmiştir:
// the first step is to create a date object instance // we cna do this by writing the following and // setting it to a variable or constant of our choice let today = new Date(); console.log("We are at year: " + today.getFullYear()); console.log("We can also get month in number:"); console.log(today.getMonth()); console.log("Also we can get the hour of the day like this:"); console.log(today.getHours()); console.log("We can also get the exact minutes along with the seconds"); console.log(today.getMinutes()); console.log(today.getSeconds()); // once we have these numbers we can use them as we like // if we want we can display them or make decisions based on them. // if we want to display month with a name // rather than a number, we can also achieve that // with the following const months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]; console.log("We are at the month of " + months[today.getMonth()]); // what we just did was to create an array to store month names // and then choose the correct month using an index value // provided by the .getMonth() method. // if we wanted to turn on dark mode after 8 pm, // we could do that with the following code // one of the first thing we should remember is that // hours are given in the 24 hour format // that means 8pm will mean 20 as hours // we can also use a short hand way // and combine the new date object creation // with the get hours method let timeOfDay = new Date().getHours(); if (timeOfDay >= 20) { console.log("Turning on Dark Mode..."); } else { console.log("Do not turn on the Dark Mode"); } // since current time is over 8pm, // in this case we expect to get turn on Dark Mode. // which is also the reuslt we get as we can see from // the console output.
Yukarıdaki kodu çalıştırmak bize aşağıdaki konsol günlüklerini verecektir:
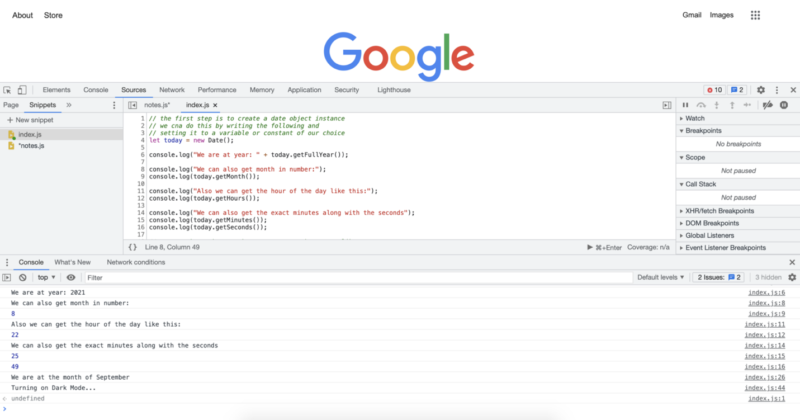
Harita() yöntemi
Harita yöntemi, size birçok kod satırı kazandırabilen ve onu nasıl kullandığınıza bağlı olarak kodunuzu çok daha temiz hale getirebilen oldukça kullanışlı bir yöntemdir. Bir dizi üzerinde döngü yapmak için kullandığınızda, esasen for döngüsünün yerini alır. İşte map() yöntemine bazı örnekler.
// lets create an array we will use for mapping let someNumbers = [1, 2, 3, 4, 5]; // lets also create the functions we will // provide to the map method function doubleNums(num1){ return num1 * 2; } function squareNums(num1){ return num1 * num1; } function add100(num1){ return num1 + 100; } console.log("Doubled numbers array:"); console.log(someNumbers.map(doubleNums)); console.log("Squared numbers array:"); console.log(someNumbers.map(squareNums)); console.log("100 added to each of the element in the umbers array:"); console.log(someNumbers.map(add100)); // map() method will loop over each of the // items in a given array and apply the // provided function // note that we do not include paranthesis // after the function names, this would call the function // instead we pass the function name, // and map() method calls them when it needs to
Yukarıdaki kodu çalıştırmak bize aşağıdaki konsol günlüklerini verecektir:

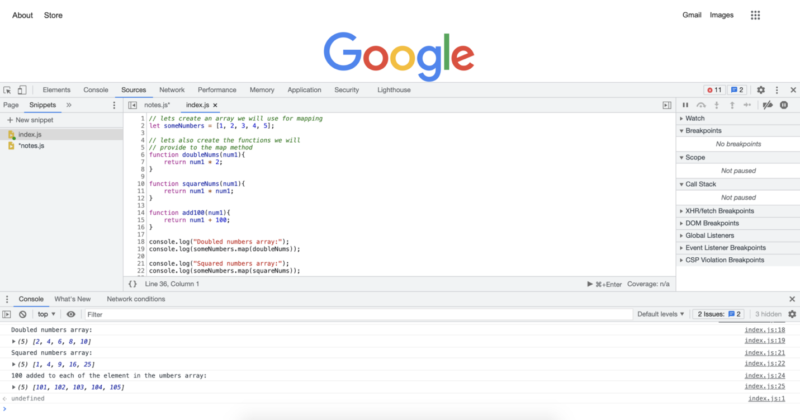
Filter() Yöntemi
map() yöntemiyle birlikte filter() yönteminin ikisi de oldukça yaygın JavaScript yöntemleridir. Az önce gördüğümüz map() yöntemine çok benziyorlar. map() yöntemiyle herhangi bir işleve geçebiliriz ve bu işlev bir dizideki öğelerin her birine uygulanır. filter() yöntemiyle, bir filtre kriteri ileteceğiz ve filtre yöntemi, bir dizideki tüm öğeler üzerinde döngü oluşturacak ve yalnızca kriterleri geçen öğeler kalacak şekilde yeni bir dizi döndürecektir. Buna birkaç örnek verelim:
// lets first create an array // to apply the filter() method let someNumbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21]; function checkEven(num1){ if (num1 % 2 == 0){ return num1; } } function checkOdd(num1){ if (num1 % 2 == 1){ return num1; } } function over13(num1){ if (num1 > 13){ return num1; } } function divisibleByFive(num){ if (num % 5 == 0){ return num; } } console.log("Even numbers from the list:"); console.log(someNumbers.filter(checkEven)); console.log("Odd numbers from the list:"); console.log(someNumbers.filter(checkOdd)); console.log("Numbers over 13 from the array:"); console.log(someNumbers.filter(over13)); console.log("Numbers divisible by 5 from the array:"); console.log(someNumbers.filter(divisibleByFive));Yukarıdaki kodu çalıştırmak bize aşağıdaki konsol günlüklerini verecektir:
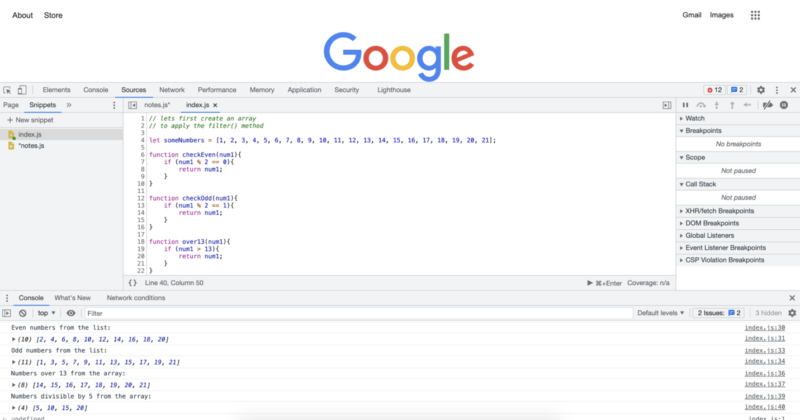
Ok işlevi
JavaScript'te işlevlerin son derece yaygın olduğunu ve daha performanslı veya temiz kod elde etmek için birçok optimizasyon yapıldığını söylediğimizi hatırlıyor musunuz? Ok fonksiyonları da bunlardan biri. Ok işlevlerine bazen şişman ok da denir. Esasen işlevlerinizi yazmak için çok daha kısa bir yol sağlarlar. Ayrıca az önce gördüğümüz JavaScript yöntemleriyle de çok yaygın olarak kullanılırlar. Bunları birkaç örnekle görelim:
// JavaScript provides multiple levels of // code shortening with arrow functions depending on your exact code // Essantially the longest way we can write a function is // the way we always write them without using the arrow functions // lets start with an array to apply the arrow functions let someNumbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21]; console.log("Original Array:"); console.log(someNumbers); // in the previous examples we have applied many functions // after creating them as regular named functions // In this example we will apply the exact transformations // so that you can see the both extremes in code lenght // double every number in the array: console.log("Double every number in the array:") console.log(someNumbers.map(num => num * 2)); // square every number in the array: console.log("Square every number in the array:") console.log(someNumbers.map(num => num * num)); // add 100 to every number in the array: console.log("Add 100 to every number in the array:") console.log(someNumbers.map(num => num + 100)); // Only keep the even numbers in the array: console.log("Only keep the even numbers in the array:") console.log(someNumbers.filter(num => num % 2 == 0)); // Only keep the odd numbers in the array: console.log("Only keep the odd numbers in the array:") console.log(someNumbers.filter(num => num % 2 == 1)); // Only keep the numbers that are evenly divisible by 5: console.log("Only keep the numbers that are evenly divisible by 5:") console.log(someNumbers.filter(num => num % 5 == 0)); // Only keep the numbers that are over 13: console.log("Only keep the numbers that are over 13:") console.log(someNumbers.filter(num => num > 13));
Yukarıdaki kodu çalıştırmak bize aşağıdaki konsol günlüklerini verecektir:
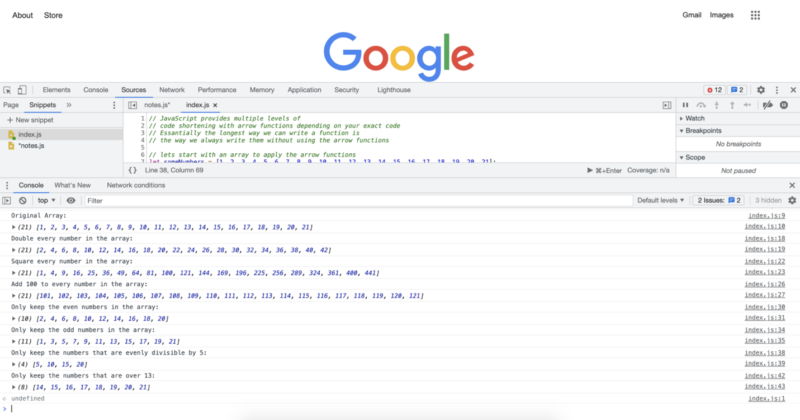
Bir sonraki derste, gördüklerimize dair bir genel bakışa sahip olacağız ve sırada ne olduğunu göreceğiz.
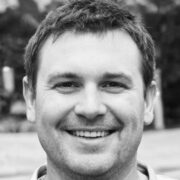
Yazar: Robert Whitney
BT departmanlarına koçluk yapan JavaScript uzmanı ve eğitmeni. Ana hedefi, başkalarına kodlama yaparken nasıl etkili bir şekilde işbirliği yapacaklarını öğreterek ekip üretkenliğini yükseltmektir.
10 blog gönderisinde Başlangıç Düzeyinden İleri Düzeye JavaScript Kursu:
- JavaScript'te kodlamaya nasıl başlanır?
- JavaScript temelleri
- JavaScript'te değişkenler ve farklı veri türleri
- Snippet'ler ve kontrol yapıları
- Döngüler ve döngüler için
- Java dizisi
- JavaScript işlevleri
- JavaScript nesneleri
- JavaScript yöntemleri ve daha fazlası
- JavaScript kursunun özeti