JavaScript 方法等等。 第 9 部分 JavaScript 課程從初學者到高級,共 10 篇博文
已發表: 2021-11-09這是 JavaScript 博客文章系列的第 9 部分,它將帶您從初學者到高級。 這次我們將探討以下主題:JavaScript 方法、默認參數值、JavaScript 中的 Date 對象和箭頭函數。 在本系列結束時,您將了解開始使用 JavaScript 編碼所需的所有基礎知識。 如果您還沒有閱讀上一篇關於 JavaScript 對象的博客文章,您可以在此處閱讀。 事不宜遲,讓我們開始第九個教程。
JavaScript 方法等 - 目錄:
- 默認參數值
- JavaScript 中的 Date 對象
- 地圖()方法
- Filter() 方法
- 箭頭函數
到目前為止,我們已經在 JavaScript 中看到了許多概念和主題,但仍有一些常用的我們沒有發現。 在本教程中,我們將了解它們的全部內容。 第一個是 JavaScript 中的默認參數值。
默認參數值
函數在編程中非常普遍,當使用某些東西時,通常不僅有像 React 這樣的框架利用 JavaScript 函數,而且還開發了進一步的優化,甚至可以從 JavaScript 函數中獲得更多。 我們在函數中擁有的主要功能之一稱為默認參數值。 默認參數允許我們編寫更安全的代碼,可以對用戶輸入做出安全的假設。 這也為用戶提供了一個默認設置,使他們更容易從他們的選項中進行選擇,從而使用戶受益。 讓我們看一些例子。
// assume you are developing a website for a coffee shop // and most people want to get a medium size americano // knowing this you want to make it easir for people to // order their coffee with less hustle function orderCoffee(typeOfCoffee="americano", sizeOfCoffee="medium", numberOfCoffee=1){ return (numberOfCoffee + " " + sizeOfCoffee + " size " + typeOfCoffee + " is being prepared. "); } console.log("Default order:"); console.log(orderCoffee()); // this way, when an average customer orders online, // it will be much easier for them to order their daily coffee // so much so that they will be able to order it with a single button // alternatively people can also customize their coffee // by changing the inputs console.log("Custom order:"); console.log(orderCoffee("latte", "large", 2)); console.log("Another custom order:"); console.log(orderCoffee("macchiato", "small", 1)); // it is also possible to change only part of the inputs // and leverage the default parameters // for the rest of the input fields console.log("Partially customized order:"); console.log(orderCoffee("iced coffee"));
運行上面的代碼會給我們以下輸出:
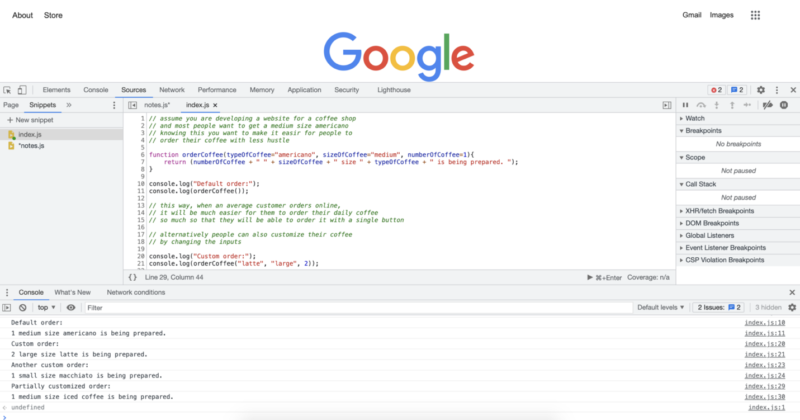
JavaScript 中的 Date 對象
JavaScript 中的 Date 對象非常常用,尤其是在 Web 開發中。 我們可以使用 Date 對象來執行時間敏感的功能,例如將顯示設置更改為暗模式、亮模式或用戶可能喜歡的任何其他模式。 我們還可以在我們正在處理的項目中根據需要使用日期信息。 以下是 Date 對象的一些示例:
// the first step is to create a date object instance // we cna do this by writing the following and // setting it to a variable or constant of our choice let today = new Date(); console.log("We are at year: " + today.getFullYear()); console.log("We can also get month in number:"); console.log(today.getMonth()); console.log("Also we can get the hour of the day like this:"); console.log(today.getHours()); console.log("We can also get the exact minutes along with the seconds"); console.log(today.getMinutes()); console.log(today.getSeconds()); // once we have these numbers we can use them as we like // if we want we can display them or make decisions based on them. // if we want to display month with a name // rather than a number, we can also achieve that // with the following const months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]; console.log("We are at the month of " + months[today.getMonth()]); // what we just did was to create an array to store month names // and then choose the correct month using an index value // provided by the .getMonth() method. // if we wanted to turn on dark mode after 8 pm, // we could do that with the following code // one of the first thing we should remember is that // hours are given in the 24 hour format // that means 8pm will mean 20 as hours // we can also use a short hand way // and combine the new date object creation // with the get hours method let timeOfDay = new Date().getHours(); if (timeOfDay >= 20) { console.log("Turning on Dark Mode..."); } else { console.log("Do not turn on the Dark Mode"); } // since current time is over 8pm, // in this case we expect to get turn on Dark Mode. // which is also the reuslt we get as we can see from // the console output.
運行上面的代碼將為我們提供以下控制台日誌:
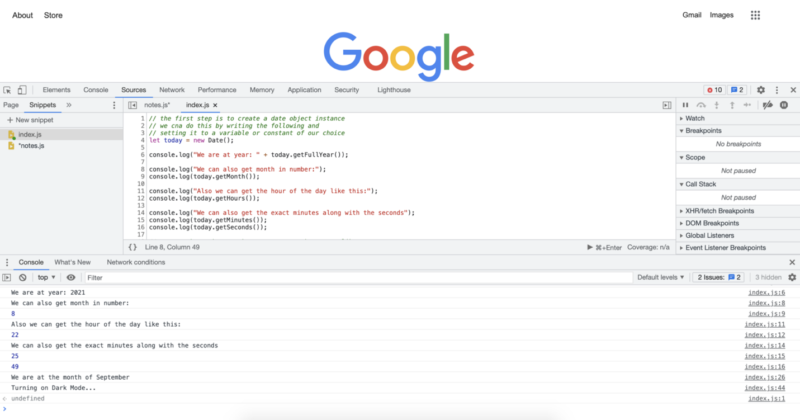
地圖()方法
map 方法是一種非常有用的方法,它可以為您節省許多代碼行,並且根據您的使用方式,它可以使您的代碼更加簡潔。 當您使用 for 循環遍歷數組時,它基本上取代了使用 for 循環。 以下是 map() 方法的一些示例。
// lets create an array we will use for mapping let someNumbers = [1, 2, 3, 4, 5]; // lets also create the functions we will // provide to the map method function doubleNums(num1){ return num1 * 2; } function squareNums(num1){ return num1 * num1; } function add100(num1){ return num1 + 100; } console.log("Doubled numbers array:"); console.log(someNumbers.map(doubleNums)); console.log("Squared numbers array:"); console.log(someNumbers.map(squareNums)); console.log("100 added to each of the element in the umbers array:"); console.log(someNumbers.map(add100)); // map() method will loop over each of the // items in a given array and apply the // provided function // note that we do not include paranthesis // after the function names, this would call the function // instead we pass the function name, // and map() method calls them when it needs to
運行上面的代碼將為我們提供以下控制台日誌:

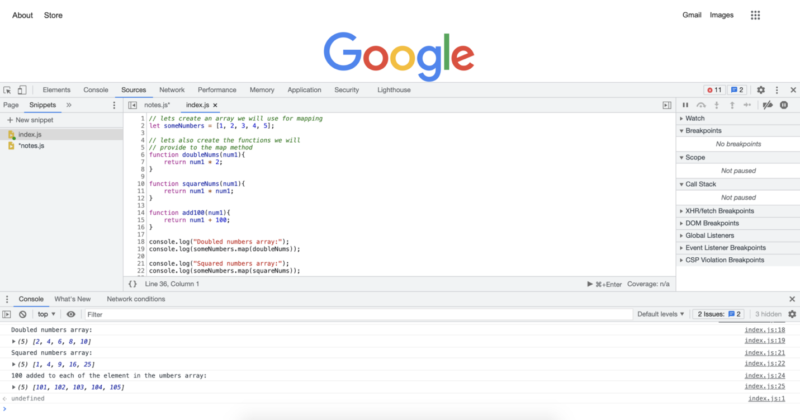
Filter() 方法
filter() 方法和 map() 方法都是非常常見的 JavaScript 方法。 它們與我們剛剛看到的 map() 方法非常相似。 使用 map() 方法,我們可以傳入任何函數,並且該函數被應用於數組中的每個元素。 使用 filter() 方法,我們將傳入一個過濾條件,該過濾器方法將遍歷數組中的所有項目,它會返回一個新數組,其中僅保留通過條件的項目。 讓我們看一些例子:
// lets first create an array // to apply the filter() method let someNumbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21]; function checkEven(num1){ if (num1 % 2 == 0){ return num1; } } function checkOdd(num1){ if (num1 % 2 == 1){ return num1; } } function over13(num1){ if (num1 > 13){ return num1; } } function divisibleByFive(num){ if (num % 5 == 0){ return num; } } console.log("Even numbers from the list:"); console.log(someNumbers.filter(checkEven)); console.log("Odd numbers from the list:"); console.log(someNumbers.filter(checkOdd)); console.log("Numbers over 13 from the array:"); console.log(someNumbers.filter(over13)); console.log("Numbers divisible by 5 from the array:"); console.log(someNumbers.filter(divisibleByFive));運行上面的代碼將為我們提供以下控制台日誌:
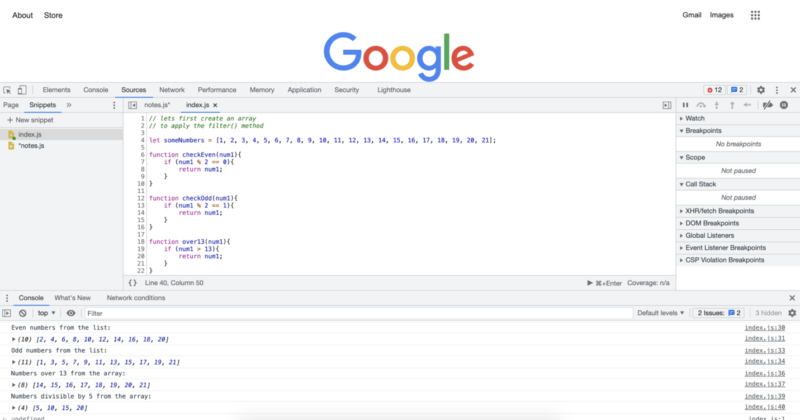
箭頭功能
還記得我們說過函數在 JavaScript 中非常常見,並且對它們進行了許多優化,甚至可以獲得更高性能或更簡潔的代碼嗎? 好吧,箭頭函數就是其中之一。 箭頭函數有時也稱為胖箭頭。 它們本質上提供了一種更短的方法來編寫你的函數。 它們也非常常用於我們剛剛看到的 JavaScript 方法。 讓我們用一些例子來看看它們:
// JavaScript provides multiple levels of // code shortening with arrow functions depending on your exact code // Essantially the longest way we can write a function is // the way we always write them without using the arrow functions // lets start with an array to apply the arrow functions let someNumbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21]; console.log("Original Array:"); console.log(someNumbers); // in the previous examples we have applied many functions // after creating them as regular named functions // In this example we will apply the exact transformations // so that you can see the both extremes in code lenght // double every number in the array: console.log("Double every number in the array:") console.log(someNumbers.map(num => num * 2)); // square every number in the array: console.log("Square every number in the array:") console.log(someNumbers.map(num => num * num)); // add 100 to every number in the array: console.log("Add 100 to every number in the array:") console.log(someNumbers.map(num => num + 100)); // Only keep the even numbers in the array: console.log("Only keep the even numbers in the array:") console.log(someNumbers.filter(num => num % 2 == 0)); // Only keep the odd numbers in the array: console.log("Only keep the odd numbers in the array:") console.log(someNumbers.filter(num => num % 2 == 1)); // Only keep the numbers that are evenly divisible by 5: console.log("Only keep the numbers that are evenly divisible by 5:") console.log(someNumbers.filter(num => num % 5 == 0)); // Only keep the numbers that are over 13: console.log("Only keep the numbers that are over 13:") console.log(someNumbers.filter(num => num > 13));
運行上面的代碼將為我們提供以下控制台日誌:
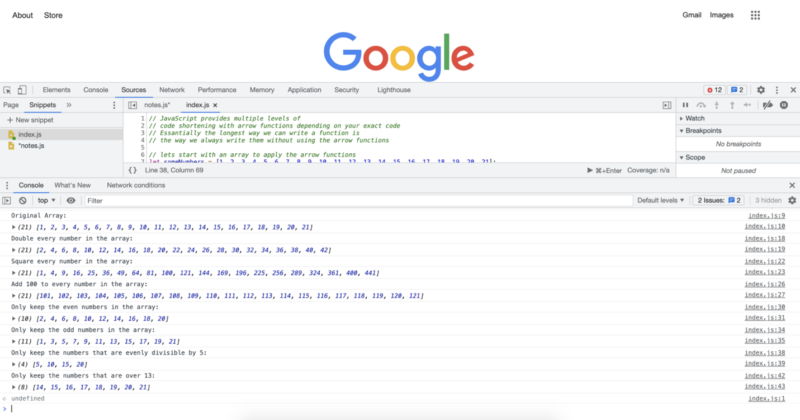
在下一個教程中,我們將概述我們已經看到的內容並看看接下來會發生什麼。
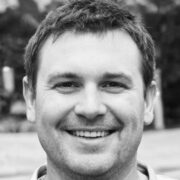
作者:羅伯特·惠特尼
JavaScript 專家和指導 IT 部門的講師。 他的主要目標是通過教其他人如何在編碼時有效合作來提高團隊生產力。
10 篇博文中從初級到高級的 JavaScript 課程:
- 如何開始用 JavaScript 編碼?
- JavaScript 基礎
- JavaScript 中的變量和不同的數據類型
- 片段和控制結構
- While 循環和 for 循環
- Java 數組
- JavaScript 函數
- JavaScript 對象
- JavaScript 方法等
- JavaScript 課程總結