實踐中的 Python 應用程序。 第 11 部分 Python 課程從初學者到高級,共 11 篇博文
已發表: 2022-01-27在這篇文章中,將幫助讀者利用之前所有博客中的知識來製作一個迷你項目。 您將在實踐中發現 Python 應用程序。 我們將使用 Visual Studio Code 作為我們的代碼編輯器。 如果您還沒有安裝 Visual Studio Code,說明在第一篇博客中給出。
實踐中的 Python 應用程序——創建一個猜數字遊戲
學習如何使用函數以及我們在之前的博客中學到的大多數其他東西,這個迷你項目將是令人興奮的。 這個迷你項目遊戲會生成一個從 1 到 1000 的隨機數,或者如果你想讓它更容易,你可以減小範圍,玩遊戲的用戶必須猜出這個數字。 聽起來很令人興奮,不是嗎? 更令人興奮的是,如果用戶猜錯了數字,我們可以給用戶一些提示,以便他們正確猜出數字。
讓我們在實踐中使用 Python 應用程序為遊戲繪製藍圖。
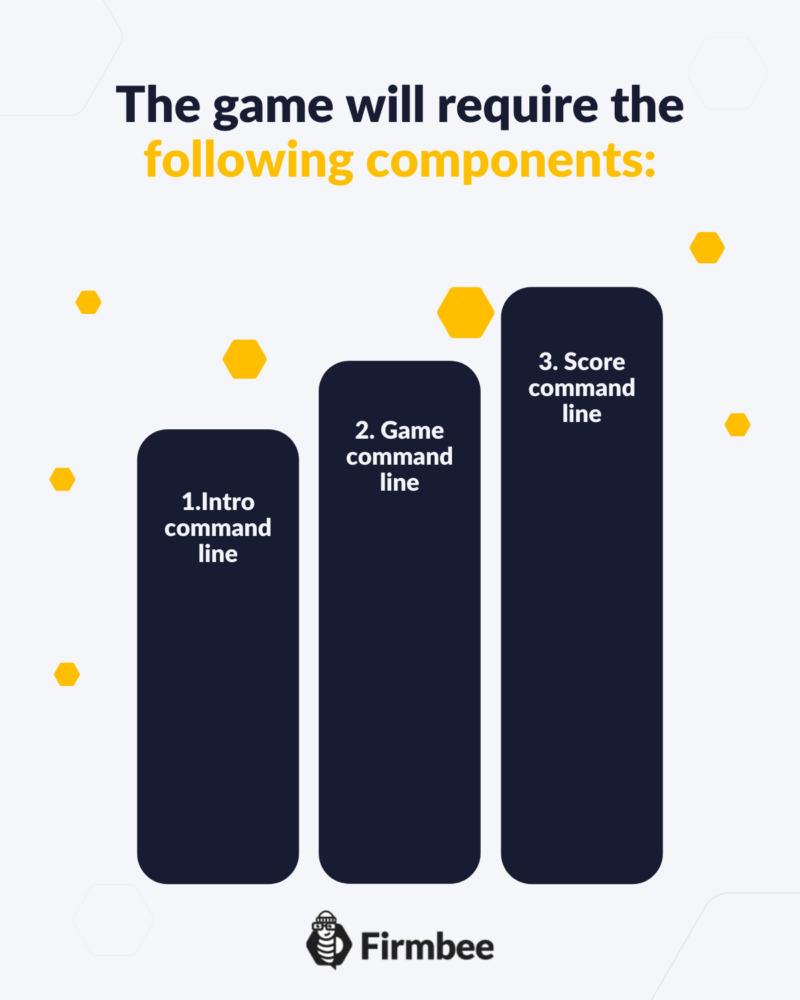
介紹命令行
在 intro 命令行中,我們將要求用戶猜測一個數字。 我們會問他的名字和年齡。 然後我們會問他是否想玩這個遊戲。 讓我們在代碼中執行此操作。
# Intro Panel Command line print("Welcome to the guessnum") name=input("what is your name?") print(f"Hello {name}")
Output: Welcome to the guessnum Hello john
可以看出,我們首先向用戶介紹了我們的遊戲,然後我們詢問了用戶的姓名。 我們使用保存的名稱向他們打招呼。 現在讓我們詢問用戶的年齡。
# Intro Panel Command line print("Welcome to the guessnum") name=input("what is your name?") age=int(input(f"Hello {name}, what is your age?")) print(f"Hello {name}")
Output: Welcome to the guessnum Hello john
在這裡,我們看到了 fstring,這是格式的替代方法,如果我們在 f 後跟一個字符串,我們可以直接在“{}”中使用我們存儲的變量。
現在我們可以看到大部分介紹面板。 現在讓我們問用戶他是否想玩遊戲,如果他想玩遊戲,讓他猜一個數字,我們可以說它是否正確。 但在讓用戶猜數字之前,我們必須準備好程序的數字。 讓我們看看它是如何在代碼中完成的。
# Intro Panel Command line print("Welcome to the guessnum") name=input("what is your name?") age=int(input(f"Hello {name}, what is your age?")) choice=input(f"Hello {name}, would you like to play the game? y/n") if choice=="y": pass else: print("exiting") exit
現在我們正在製作另一個提示,詢問用戶他是否想玩遊戲,如果他說是,我們將使用我們在以前的博客中學到的條件繼續,如果不是,則退出遊戲。 現在讓我們繼續擴展我們的遊戲並向用戶詢問數字,但在此之前讓我們的代碼選擇一個隨機數。
# Intro Panel Command line import random print("Welcome to the guessnum") name=input("what is your name?") age=int(input(f"Hello {name}, what is your age?")) choice=input(f"Hello {name}, would you like to play the game? y/n") if choice=="y": number=int(random.randint(1,5)) guess=int(input("Please input your guess")) print(f"your guess is {guess}") else: print("exiting") exit
Output: Welcome to the guessnum your guess is 2
現在我們添加了一個名為 random 的導入,它從給定範圍中選擇一個隨機數。 函數是 random.randint(start,end)。 然後我們要求我們的用戶猜測這個數字,我們正在打印我們的用戶猜測。
讓我們也打印程序的猜測。
# Intro Panel Command line import random print("Welcome to the guessnum") name=input("what is your name?") age=int(input(f"Hello {name}, what is your age?")) choice=input(f"Hello {name}, would you like to play the game? y/n") if choice=="y": number=int(random.randint(1,5)) guess=int(input("Please input your guess")) print(f"your guess is {guess} and program's guess is {number}") else: print("exiting") exit
output: Welcome to the guessnum your guess is 2 and the program's guess is 5
所以,我們可以看到,我們已經快到一半了,我們有了程序的猜測和用戶的猜測。 現在我們可以比較並打印用戶是否正確。
# Intro Panel Command line import random print("Welcome to the guessnum") name=input("what is your name?") age=int(input(f"Hello {name}, what is your age?")) choice=input(f"Hello {name}, would you like to play the game? y/n") if choice=="y": number=int(random.randint(1,5)) guess=int(input("Please input your guess")) if guess==number: print("you guessed it right!!!") print(f"your guess is {guess} and program's guess is {number}. Sorry!!! your guess is wrong") else: print("exiting") exit
output: Welcome to the guessnum your guess is 2 and the program's guess is 1. Sorry!!! your guess is wrong
如您所見,我猜錯了,也許您可以猜對。 通過添加分數因素,可以使這個遊戲變得更有趣。 現在讓我們為分數因子編寫代碼。

# Intro Panel Command line import random print("Welcome to the guessnum") name=input("what is your name?") age=int(input(f"Hello {name}, what is your age?")) choice=input(f"Hello {name}, would you like to play the game? y/n") correct=0 while(choice=="y"): number=int(random.randint(1,5)) guess=int(input("Please input your guess")) if guess==number: print("you guessed it right!!!") correct+=1 choice=input(f"Hello {name}, would you like to continue the game? y/n") print(f"your guess is {guess} and program's guess is {number}. Sorry!!! your guess is wrong") choice=input(f"Hello {name}, would you like to continue the game? y/n") else: print(f"your score is {correct}") print("exiting") exit
output: Welcome to the guessnum your guess is 1 and program's guess is 5. Sorry!!! your guess is wrong your guess is 2 and program's guess is 3. Sorry!!! your guess is wrong your guess is 3 and program's guess is 2. Sorry!!! your guess is wrong your guess is 4 and program's guess is 3. Sorry!!! your guess is wrong your guess is 1 and program's guess is 2. Sorry!!! your guess is wrong your guess is 2 and program's guess is 5. Sorry!!! your guess is wrong your guess is 3 and program's guess is 4. Sorry!!! your guess is wrong your guess is 3 and program's guess is 2. Sorry!!! your guess is wrong your guess is 3 and program's guess is 5. Sorry!!! your guess is wrong your guess is 4 and program's guess is 2. Sorry!!! your guess is wrong your guess is 3 and program's guess is 1. Sorry!!! your guess is wrong your guess is 4 and program's guess is 5. Sorry!!! your guess is wrong your guess is 2 and program's guess is 2. you guessed it right!!! Sorry!!! your guess is wrong your score is 1 exiting
如您所見,我們使用了 while 循環,並使用了一個名為正確的新變量,它為我們提供了用戶的分數。 我們正在打印到輸出。
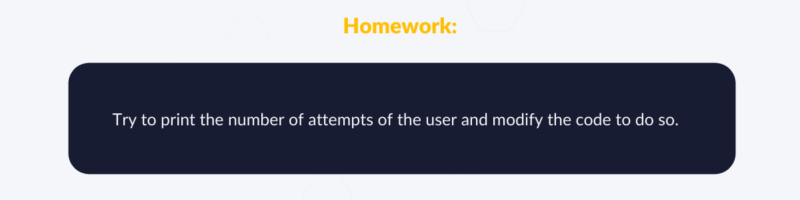
您可能還喜歡我們從初級到高級的 JavaScript 課程。
恭喜! 現在您知道如何將 Python 應用程序付諸實踐,並且您正式完成了課程:Python Course from Beginner to Advanced in 11 篇博文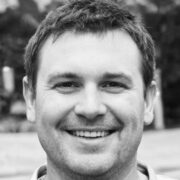
作者:羅伯特·惠特尼
JavaScript 專家和指導 IT 部門的講師。 他的主要目標是通過教其他人如何在編碼時有效合作來提高團隊生產力。
11 篇博文中從初級到高級的 Python 課程:
- Python課程簡介。 第 1 部分 Python 課程從初學者到高級,共 11 篇博文
- Python 中的變量和數據類型。 第 2 部分 Python 課程從初學者到高級,共 11 篇博文
- Python 元組、列表、集合和字典。 第 3 部分 Python 課程從初學者到高級,共 11 篇博文
- Python 集和字典。 第 4 部分 Python 課程從初學者到高級,共 11 篇博文
- Python 中的條件語句。 第 5 部分 Python 課程從初學者到高級,共 11 篇博文
- Python中的循環。 第 6 部分 Python 課程從初學者到高級,共 11 篇博文
- Python 函數。 第 7 部分 Python 課程從初學者到高級,共 11 篇博文
- Python 中的高級函數。 第 8 部分 Python 課程從初學者到高級,共 11 篇博文
- Python 類和對象。 第 9 部分 Python 課程從初學者到高級,共 11 篇博文
- Python 中的文件。 第 10 部分 Python 課程從初學者到高級,共 11 篇博文
- 實踐中的 Python 應用程序。 第 11 部分 Python 課程從初學者到高級,共 11 篇博文